在qml的listview中如何使用QAbstractItemModel
时间: 2024-04-29 08:23:37 浏览: 138
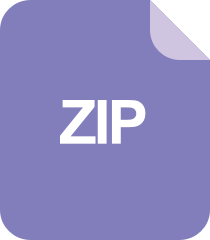
SideBar:SideBar通过ListView标头
在QML中使用QAbstractItemModel需要通过C++代码编写自定义的QAbstractItemModel子类。下面是一个简单的示例:
1. 编写自定义的QAbstractItemModel子类
```
// mymodel.h
#ifndef MYMODEL_H
#define MYMODEL_H
#include <QAbstractItemModel>
class MyModel : public QAbstractItemModel
{
Q_OBJECT
public:
explicit MyModel(QObject *parent = nullptr);
// 获取模型数据的数量和索引
int rowCount(const QModelIndex &parent = QModelIndex()) const override;
int columnCount(const QModelIndex &parent = QModelIndex()) const override;
QModelIndex index(int row, int column, const QModelIndex &parent = QModelIndex()) const override;
// 获取模型数据
QVariant data(const QModelIndex &index, int role = Qt::DisplayRole) const override;
private:
QStringList m_data;
};
#endif // MYMODEL_H
```
```
// mymodel.cpp
#include "mymodel.h"
MyModel::MyModel(QObject *parent)
: QAbstractItemModel(parent)
{
// 初始化模型数据
m_data << "Item 1" << "Item 2" << "Item 3";
}
int MyModel::rowCount(const QModelIndex &parent) const
{
if (parent.isValid())
return 0;
return m_data.count();
}
int MyModel::columnCount(const QModelIndex &parent) const
{
if (parent.isValid())
return 0;
return 1;
}
QModelIndex MyModel::index(int row, int column, const QModelIndex &parent) const
{
if (parent.isValid() || row < 0 || row >= m_data.count() || column < 0 || column >= 1)
return QModelIndex();
return createIndex(row, column);
}
QVariant MyModel::data(const QModelIndex &index, int role) const
{
if (!index.isValid())
return QVariant();
if (role == Qt::DisplayRole || role == Qt::EditRole)
return m_data.at(index.row());
return QVariant();
}
```
2. 在QML中使用自定义的QAbstractItemModel子类
```
// main.qml
import QtQuick 2.0
import QtQuick.Controls 2.0
ApplicationWindow {
visible: true
width: 400
height: 400
// 创建自定义模型
MyModel {
id: myModel
}
// 使用ListView显示模型数据
ListView {
anchors.fill: parent
model: myModel
delegate: Text {
text: model.display
}
}
}
```
阅读全文
相关推荐
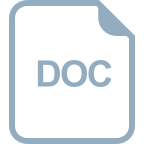
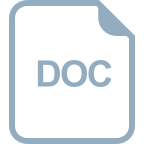
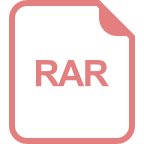








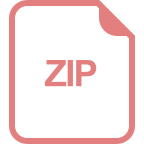
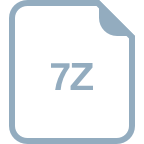
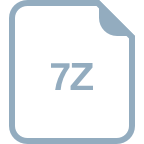
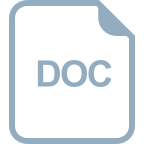
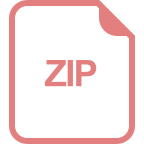
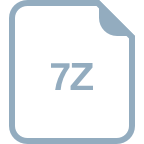
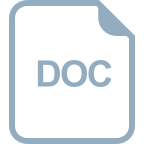