map<int,Point2d>按照x坐标从小到大重新排序
时间: 2024-12-22 20:28:43 浏览: 10
要对`std::map<int, Point2d>`中的元素按照`x`坐标从小到大进行排序,`map`本身并不支持直接排序,因为它是关联容器,而不是有序容器。但是你可以创建一个新的有序容器,比如`std::vector`或自定义的`std::multimap`,然后将`map`的内容复制过去并按照`x`坐标排序。
以下是一个使用`std::vector`的示例:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
struct Point2d {
int x;
// 其他成员...
bool operator<(const Point2d& other) const { return x < other.x; }
};
void sort_map_by_x(map<int, Point2d>& original_map, std::vector<Point2d>& sorted_points) {
for (const auto& pair : original_map) {
sorted_points.push_back(pair.second);
}
std::sort(sorted_points.begin(), sorted_points.end());
}
// 示例用法
int main() {
map<int, Point2d> original_map = ...; // 填充你的map
std::vector<Point2d> sorted_points;
sort_map_by_x(original_map, sorted_points);
// 现在sorted_points就是按x坐标从小到大排列的
for (const auto& point : sorted_points) {
std::cout << "X: " << point.x << ", Other details: ... \n";
}
return 0;
}
```
在这个例子中,我们首先遍历原`map`,将`Point2d`对象添加到`vector`中,然后使用`std::sort`函数根据`<`运算符(这里定义为`x`坐标小于其他坐标)对`vector`进行排序。
阅读全文
相关推荐



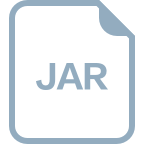
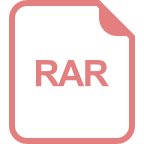










