我想要python使用threading库实现的代码
时间: 2023-03-28 11:02:00 浏览: 125
你好,以下是使用 threading 库实现的 Python 代码:
```python
import threading
def worker():
print("Thread started: {}".format(threading.current_thread().name))
# do some work here
print("Thread finished: {}".format(threading.current_thread().name))
if __name__ == '__main__':
threads = []
for i in range(5):
t = threading.Thread(target=worker)
threads.append(t)
t.start()
for t in threads:
t.join()
print("All threads finished")
```
希望能对你有所帮助。
相关问题
Python的Threading库 怎么停止某一个线程
可以使用Threading库中的Event对象来控制线程的停止。在要停止的线程中增加一个Event对象并循环检测该对象的状态,当该对象被设置为True后,线程就会停止。具体实现可以参考以下代码:
```python
import threading
class MyThread(threading.Thread):
def __init__(self, event):
threading.Thread.__init__(self)
self.stopped = event
def run(self):
while not self.stopped.wait(1):
# 执行线程的操作
print("thread is running...")
if __name__ == '__main__':
stop_event = threading.Event()
t = MyThread(stop_event)
t.start()
# 停止线程
stop_event.set()
```
在上述代码中,定义了一个MyThread类并重载了它的run方法,在run方法中增加了一个while循环,每次循环会检测Event对象的状态,如果状态为True就退出循环,从而停止该线程。在主程序中创建了一个Event对象,并创建了一个线程实例并启动了线程。当需要停止该线程时,设置Event对象的状态为True即可。
python threading_python中如何安装threading
`threading` 是 Python 标准库中的一个模块,通常情况下无需安装。如果你使用的是 Python 2.x 版本,那么 `threading` 模块已经默认安装在你的 Python 中。如果你使用的是 Python 3.x 版本,那么 `threading` 模块同样也已经默认安装在你的 Python 中。
如果你需要使用 `threading` 模块,只需要在你的 Python 代码中导入即可:
```python
import threading
```
如果你使用的是 Python 2.x 版本,你也可以使用 `thread` 模块来实现多线程编程。但是在 Python 3.x 版本中,`thread` 模块已经被废弃,建议使用 `threading` 模块来进行多线程编程。
阅读全文
相关推荐
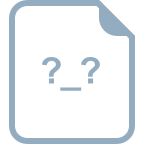
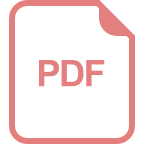
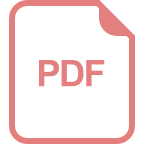
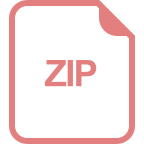
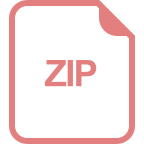
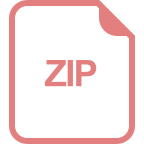
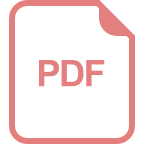
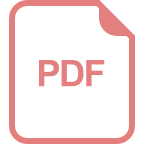
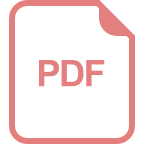
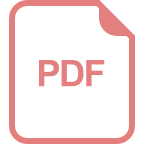
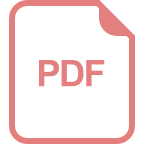
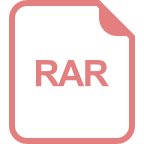
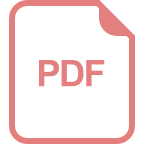
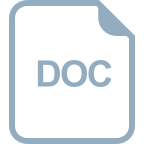
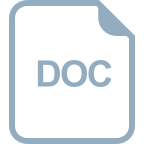
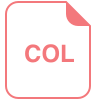