python语言编写一个生成九宫格图片的代码
时间: 2024-03-03 07:47:05 浏览: 152
好的,以下是一个生成九宫格图片的Python代码示例:
```python
from PIL import Image, ImageDraw
# 设置每个小格子的大小和九宫格的边长
cell_size = 50
grid_size = cell_size * 3
# 创建空白图像,并创建画布对象
img = Image.new('RGB', (grid_size, grid_size), color='white')
draw = ImageDraw.Draw(img)
# 绘制九宫格的线条
for i in range(2):
line_pos = (cell_size * (i + 1), 0, cell_size * (i + 1), grid_size)
draw.line(line_pos, fill='black')
line_pos = (0, cell_size * (i + 1), grid_size, cell_size * (i + 1))
draw.line(line_pos, fill='black')
# 保存生成的九宫格图片
img.save('sudoku.png')
```
这段代码使用了Python的Pillow库来创建和操作图片。它首先设置了每个小格子的大小和九宫格的边长,然后创建了一个空白的图像,并创建了一个画布对象。接着,使用循环来绘制九宫格的线条,最后保存生成的九宫格图片。你可以根据需要对这段代码进行修改和扩展。
相关问题
请帮我用python语言编写关于给定九宫格的初始状态,要求在有限步的操作内,使其转化为目标状态,且所得到的解是代价最小解(即移动的步数最少)。的代码,要求:输入是九宫格的初始状态和目标状态,输出是重排的过程,即途径的状态。
这是一个典型的回溯搜索(Backtracking Search)或A*搜索(A* Algorithm)的问题,通常用于解决迷宫问题或八皇后问题。这里我会给出一个基础的A*算法的Python实现框架,用于找到从初始状态到目标状态的最低步数路径。请注意,这个代码示例并未包含完整的解决方案,因为A*算法涉及到启发式函数和优先队列,需要额外的代码支持。
```python
import heapq
# 定义一个表示九宫格的状态类
class GridState:
def __init__(self, state, steps=0):
self.state = state
self.steps = steps
# 用启发式函数估算剩余步骤,此处假设所有元素之间的距离相等
self.heuristic = sum(abs(i - j) for i, j in zip(self.state, target_state))
def __lt__(self, other):
return self.total_cost() < other.total_cost()
def a_star_search(start_state, target_state):
open_set = []
heapq.heappush(open_set, (start_state.heuristic + start_state.steps, start_state))
while open_set:
current = heapq.heappop(open_set)
if current.state == target_state:
return generate_path(current)
for neighbor in generate_neighbors(current.state):
new_steps = current.steps + 1
total_cost = new_steps + neighbor.heuristic
heapq.heappush(open_set, (total_cost, GridState(neighbor, new_steps)))
return None
def generate_neighbors(state):
# 这里需要定义如何生成邻居状态,比如旋转、平移等操作
# 对于九宫格,可以考虑9个可能的方向变化
pass
def generate_path(end_state):
path = [end_state]
parent = end_state
while parent is not None:
path.append(parent.state)
parent = parent.previous_state # 假设每个状态都有parent信息
path.reverse()
return path
# 使用示例
start_state = ... # 初始化九宫格状态
target_state = ... # 目标九宫格状态
path = a_star_search(start_state, target_state)
if path:
print("最短路径:", path)
else:
print("无法从初始状态达到目标状态")
```
在这个例子中,你需要补充`generate_neighbors`函数来处理九宫格的各种邻接状态,并在GridState类中添加`previous_state`属性以便追踪路径。A*算法的核心在于启发式函数的选择,这取决于实际问题的具体规则。
python九宫格算术
Python九宫格算术是一种基于Python语言的算法,用于生成一个n*n的矩阵,使得矩阵中每行、每列和对角线上的数字之和相等。这个算法的实现过程中,需要使用到Python的基础语法、循环、条件判断、数组等知识,同时也可以使用Python中的库来简化代码的编写。其中,引用中的代码就是一个使用numpy库实现九宫格算术的例子。通过这个例子,我们可以看到,九宫格算术的实现过程中,需要先填充最大的数,然后按照一定的规则填充其他的数字,最终生成一个符合要求的矩阵。
阅读全文
相关推荐
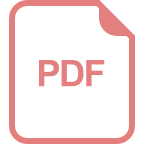
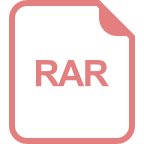
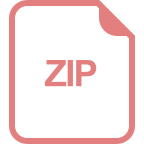
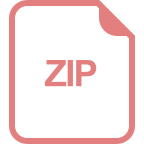
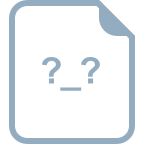
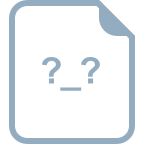
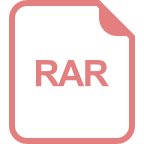
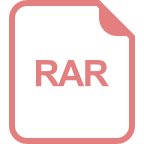
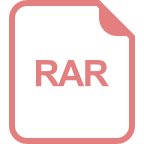
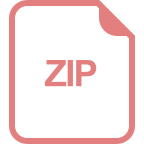
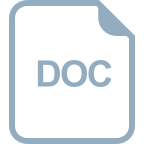
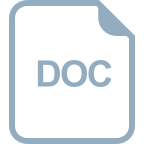
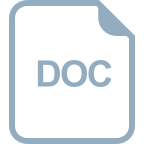