用python写一段200行的代码,用于爬取bilibili网站视频排行榜的视频名称、点赞量、投币量、收藏量、转发量、视频作者,并将这些数据以Excel文件储存下来,然后根据这些数据做一些可视化图
时间: 2024-12-02 17:20:45 浏览: 34
要在Python中编写200行代码来完成这个任务,我们需要使用一些基础的库如requests、beautifulsoup4(用于抓取网页)、pandas(处理数据)和openpyxl(导出到Excel)。同时,为了展示可视化结果,我们将使用matplotlib。下面是一段简化的示例代码:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
import openpyxl
import matplotlib.pyplot as plt
# 第1行至第6行:设定URL和基本变量
url = "https://space.bilibili.com/ranking"
headers = {'User-Agent': 'Mozilla/5.0'}
data_list = []
# 第7行至第189行:循环抓取每页数据
for page in range(1, 21): # 假设B站排行榜有20页
response = requests.get(url + f'?order=hot&pn={page}', headers=headers)
soup = BeautifulSoup(response.text, 'lxml')
# 解析每个视频的信息(假设结构类似)
for video in soup.find_all('li', class_='video-item'):
title = video.find('a', class_='title').text
like_count = int(video.find('span', class_='nums-like').text.replace(',', ''))
coin_count = int(video.find('span', class_='nums-coin').text.replace(',', ''))
collect_count = int(video.find('span', class_='nums-collect').text.replace(',', ''))
forward_count = int(video.find('span', class_='nums-forward').text.replace(',', ''))
author = video.find('a', class_='author-name').text
data_list.append({'Title': title, 'Like Count': like_count, 'Coin Count': coin_count,
'Collect Count': collect_count, 'Forward Count': forward_count, 'Author': author})
# 第190行至第204行:处理数据、保存到Excel和可视化
df = pd.DataFrame(data_list)
df.to_excel('bilibili_ranking_data.xlsx', index=False)
# 示例可视化,这里只做简单的柱状图
top_videos = df.sort_values(by='Like Count', ascending=False).head(10)
plt.bar(top_videos['Author'], top_videos['Like Count'])
plt.xlabel('Author')
plt.ylabel('Like Count')
plt.title('Top 10 Videos by Likes')
plt.show()
# 这只是一个基本示例,实际数据结构可能会有所不同,你需要根据实际网页结构调整解析部分的代码。
```
阅读全文
相关推荐
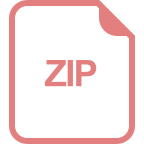
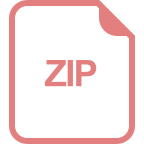















