写一个jquery轮播图
时间: 2023-11-18 10:55:35 浏览: 84
下面是一个简单的 jQuery 轮播图实现:
HTML 代码:
```html
<div class="slider">
<div class="slider-wrapper">
<div class="slider-item"><img src="image1.jpg"></div>
<div class="slider-item"><img src="image2.jpg"></div>
<div class="slider-item"><img src="image3.jpg"></div>
</div>
<div class="slider-controls">
<a href="#" class="prev">Prev</a>
<a href="#" class="next">Next</a>
</div>
</div>
```
CSS 代码:
```css
.slider {
position: relative;
width: 600px;
height: 400px;
overflow: hidden;
}
.slider-wrapper {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
}
.slider-item {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
display: none;
}
.slider-item:first-child {
display: block;
}
.slider-controls {
position: absolute;
bottom: 20px;
left: 50%;
transform: translateX(-50%);
text-align: center;
}
.slider-controls a {
display: inline-block;
margin: 0 10px;
padding: 10px 20px;
background-color: #333;
color: #fff;
text-decoration: none;
border-radius: 5px;
}
.slider-controls a:hover {
background-color: #555;
}
```
jQuery 代码:
```js
$(function() {
var $wrapper = $('.slider-wrapper');
var $items = $('.slider-item');
var $prev = $('.prev');
var $next = $('.next');
var currentIndex = 0;
var intervalId;
function showItem(index) {
$items.eq(currentIndex).fadeOut();
$items.eq(index).fadeIn();
currentIndex = index;
}
$prev.on('click', function(e) {
e.preventDefault();
var index = currentIndex - 1;
if (index < 0) {
index = $items.length - 1;
}
showItem(index);
});
$next.on('click', function(e) {
e.preventDefault();
var index = currentIndex + 1;
if (index >= $items.length) {
index = 0;
}
showItem(index);
});
function startInterval() {
intervalId = setInterval(function() {
var index = currentIndex + 1;
if (index >= $items.length) {
index = 0;
}
showItem(index);
}, 3000);
}
function stopInterval() {
clearInterval(intervalId);
}
startInterval();
$wrapper.on('mouseenter', function() {
stopInterval();
});
$wrapper.on('mouseleave', function() {
startInterval();
});
});
```
这个轮播图的实现思路是,使用绝对定位将轮播项层叠在一起,然后通过控制当前轮播项的透明度来实现轮播效果。同时,通过定时器控制轮播的间隔时间,并且支持鼠标悬停时暂停轮播的功能。
阅读全文
相关推荐







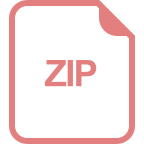







