java 根据ip获取归属地
时间: 2023-08-13 12:07:48 浏览: 68
您好,要根据 IP 地址获取归属地,可以使用 Java 自带的 `InetAddress` 类和 `ip138` 等在线查询工具。
以下是一个示例代码:
```java
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.InetAddress;
import java.net.URL;
import java.net.URLConnection;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class IPUtils {
/**
* 根据 IP 地址获取归属地
*
* @param ip IP 地址
* @return 归属地字符串
* @throws IOException
*/
public static String getIpAddress(String ip) throws IOException {
InetAddress inetAddress = InetAddress.getByName(ip);
String hostAddress = inetAddress.getHostAddress();
String url = "http://www.ip138.com/ips138.asp?ip=" + hostAddress + "&action=2";
URLConnection connection = new URL(url).openConnection();
BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream(), "gbk"));
StringBuilder stringBuilder = new StringBuilder();
String line = null;
while ((line = reader.readLine()) != null) {
stringBuilder.append(line);
}
reader.close();
String content = stringBuilder.toString();
String regex = "<li>本站主数据:(.*?)</li>";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(content);
if (matcher.find()) {
return matcher.group(1);
}
return "未知";
}
public static void main(String[] args) throws IOException {
String ip = "113.96.208.44";
String address = getIpAddress(ip);
System.out.println(ip + " 的归属地是:" + address);
}
}
```
该代码通过 `InetAddress` 类获取 IP 地址的主机名,然后构造查询 URL,请求 IP138 等在线查询工具获取归属地信息,并使用正则表达式从响应内容中提取出归属地。注意需要设置读取响应的字符编码为 GBK。
需要注意的是,该方法仅供学习和参考,实际应用中可能存在 IP 地址和归属地不匹配的情况,也可能存在在线查询工具挂掉或者响应超时的情况,需要根据实际情况进行处理。
相关推荐
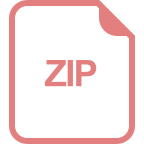
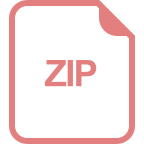
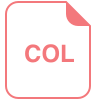
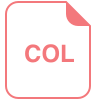












