如何利用beautifulsoup库找表格
时间: 2024-03-27 09:35:09 浏览: 16
您可以使用BeautifulSoup库中的find()或find_all()方法来找到表格,具体步骤如下:
1. 导入BeautifulSoup库和requests库
```python
from bs4 import BeautifulSoup
import requests
```
2. 使用requests库获取网页内容
```python
url = 'http://example.com'
response = requests.get(url)
```
3. 将网页内容传递给BeautifulSoup对象,并指定解析器
```python
soup = BeautifulSoup(response.text, 'html.parser')
```
4. 使用find()或find_all()方法找到表格
```python
table = soup.find('table')
```
或者
```python
tables = soup.find_all('table')
```
5. 对找到的表格进行进一步处理,例如遍历表格中的行和列,获取表格中的数据
```python
for row in table.find_all('tr'):
for cell in row.find_all('td'):
print(cell.text)
```
注意,以上步骤中的代码仅作为示例,您需要根据实际情况进行适当修改。
相关问题
beautifulsoup爬取网页表格
好的,下面是使用BeautifulSoup爬取网页表格的一般流程:
1. 导入需要的库:`requests`和`bs4`。
``` python
import requests
from bs4 import BeautifulSoup
```
2. 使用`requests`库发送请求获取页面内容。
``` python
url = "https://www.example.com/table"
response = requests.get(url)
html = response.text
```
3. 使用`BeautifulSoup`库解析页面,找到需要的表格。
``` python
soup = BeautifulSoup(html, 'html.parser')
table = soup.find('table', {'class': 'table_class'})
```
4. 遍历表格中的行和列,将数据存储到列表中。
``` python
data = []
for row in table.find_all('tr'):
row_data = []
for cell in row.find_all('td'):
row_data.append(cell.text.strip())
data.append(row_data)
```
5. 对于需要的数据进行处理,保存到文件或数据库中。
以上就是使用BeautifulSoup爬取网页表格的一般流程,具体的代码实现可以根据实际情况进行调整。
BeautifulSoup获取表格
你可以使用BeautifulSoup来获取网页上的表格数据。首先,你需要导入BeautifulSoup库并从网络上获取HTML页面。然后,你可以使用BeautifulSoup的find()或find_all()方法来找到表格标签(<table>),并进一步处理其中的行和列。
以下是一个使用BeautifulSoup获取表格数据的示例代码:
```python
import requests
from bs4 import BeautifulSoup
# 从网页中获取HTML数据
url = 'https://www.example.com' # 替换为你要抓取的网页URL
response = requests.get(url)
html = response.text
# 使用BeautifulSoup解析HTML
soup = BeautifulSoup(html, 'html.parser')
# 找到表格标签
table = soup.find('table')
# 遍历表格的行和列
for row in table.find_all('tr'):
cells = row.find_all('td')
for cell in cells:
print(cell.text) # 打印每个单元格的文本内容
```
在上面的示例中,我们使用了requests库来从网页中获取HTML数据,并使用BeautifulSoup库来解析HTML。我们通过调用`find()`方法找到第一个符合条件的表格标签,然后使用`find_all()`方法找到其中的行和列。最后,我们遍历每个单元格,并打印其文本内容。
请记得将示例中的URL替换为你要抓取的网页的真实URL。此外,你可能还需要根据实际情况调整代码以适应不同的表格结构。
相关推荐
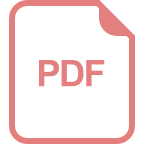
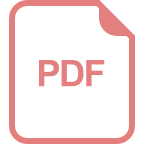
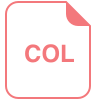
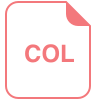
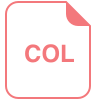









