java数独计算
时间: 2023-10-09 19:17:21 浏览: 49
Java实现数独计算可以使用回溯算法,以下是一个简单的实现:
```java
public class SudokuSolver {
private static final int SIZE = 9; // 数独大小
private static final int EMPTY = 0; // 空格子的标志
// 判断数独的某一行是否合法
private static boolean isValidRow(int[][] board, int row, int num) {
for (int col = 0; col < SIZE; col++) {
if (board[row][col] == num) {
return false;
}
}
return true;
}
// 判断数独的某一列是否合法
private static boolean isValidCol(int[][] board, int col, int num) {
for (int row = 0; row < SIZE; row++) {
if (board[row][col] == num) {
return false;
}
}
return true;
}
// 判断数独的某一宫是否合法
private static boolean isValidBox(int[][] board, int boxStartRow, int boxStartCol, int num) {
for (int row = 0; row < 3; row++) {
for (int col = 0; col < 3; col++) {
if (board[row + boxStartRow][col + boxStartCol] == num) {
return false;
}
}
}
return true;
}
// 判断某个位置是否可以填入指定的数字
private static boolean isValidEntry(int[][] board, int row, int col, int num) {
return isValidRow(board, row, num)
&& isValidCol(board, col, num)
&& isValidBox(board, row - row % 3, col - col % 3, num);
}
// 使用回溯算法解决数独
public static boolean solveSudoku(int[][] board) {
for (int row = 0; row < SIZE; row++) {
for (int col = 0; col < SIZE; col++) {
if (board[row][col] == EMPTY) {
for (int num = 1; num <= SIZE; num++) {
if (isValidEntry(board, row, col, num)) {
board[row][col] = num;
if (solveSudoku(board)) {
return true;
} else {
board[row][col] = EMPTY;
}
}
}
return false;
}
}
}
return true;
}
}
```
在上述代码中,isValidRow、isValidCol、isValidBox分别用于判断某一行、某一列、某一宫是否合法,isValidEntry用于判断某个位置是否可以填入指定的数字,solveSudoku是使用回溯算法解决数独的核心方法。
相关推荐
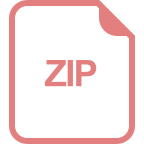
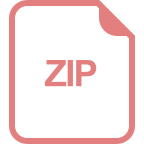














