数独java保存文件
时间: 2024-05-20 17:09:51 浏览: 59
在Java中,数独保存文件可以使用文本文件或二进制文件的形式进行保存。常见的保存文件格式有txt、csv等,其中txt格式比较常用。可以通过Java中的FileWriter或BufferedWriter来实现保存txt格式文件的操作。另外,如果要保存二进制文件,可以使用Java中的ObjectOutputStream类来实现。这个类可以将对象序列化并输出到文件中,以便之后读取。
在保存数独时,可以将每个数字保存为一个字符或数字,并用分隔符隔开每个数字。例如,可以使用逗号作为分隔符将每个数字保存在同一行中。另外,在保存时应该同时保存数独的难度级别和解答状态,这样在之后读取时可以根据需要进行过滤和筛选。
相关问题
用Java swing gui 实现数独游戏的代码,要求1、要求产生指定形式的数独题目 2、在产生的数独题目上,程序产生数独的答案,将题目和答案保存在文件。 3、用户可按指定格式输入数独答案,程序可以检查答案是否正确,如
下面是一个简单的 Java Swing GUI 实现数独游戏的代码:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.io.*;
public class SudokuGame extends JFrame {
private JPanel gamePanel;
private JPanel buttonPanel;
private JButton newGameButton;
private JButton checkButton;
private JButton saveButton;
private JButton loadButton;
private JTextField[][] cells;
private int[][] solution;
private int[][] puzzle;
public SudokuGame() {
super("Sudoku Game");
// Create the game panel
gamePanel = new JPanel(new GridLayout(9, 9));
cells = new JTextField[9][9];
for (int i = 0; i < 9; i++) {
for (int j = 0; j < 9; j++) {
cells[i][j] = new JTextField();
cells[i][j].setHorizontalAlignment(JTextField.CENTER);
cells[i][j].setFont(new Font("Arial", Font.PLAIN, 20));
gamePanel.add(cells[i][j]);
}
}
// Create the button panel
buttonPanel = new JPanel(new GridLayout(1, 4));
newGameButton = new JButton("New Game");
newGameButton.addActionListener(new NewGameListener());
checkButton = new JButton("Check");
checkButton.addActionListener(new CheckListener());
saveButton = new JButton("Save");
saveButton.addActionListener(new SaveListener());
loadButton = new JButton("Load");
loadButton.addActionListener(new LoadListener());
buttonPanel.add(newGameButton);
buttonPanel.add(checkButton);
buttonPanel.add(saveButton);
buttonPanel.add(loadButton);
// Add the panels to the frame
add(gamePanel, BorderLayout.CENTER);
add(buttonPanel, BorderLayout.SOUTH);
// Set the size and visibility of the frame
setSize(500, 500);
setVisible(true);
}
private boolean generatePuzzle() {
solution = generateSolution();
puzzle = new int[9][9];
for (int i = 0; i < 9; i++) {
for (int j = 0; j < 9; j++) {
puzzle[i][j] = solution[i][j];
}
}
int count = 0;
while (count < 40) {
int i = (int) (Math.random() * 9);
int j = (int) (Math.random() * 9);
if (puzzle[i][j] != 0) {
puzzle[i][j] = 0;
count++;
}
}
return solvePuzzle(puzzle);
}
private int[][] generateSolution() {
int[][] board = new int[9][9];
solveBoard(board, 0, 0);
return board;
}
private boolean solvePuzzle(int[][] puzzle) {
int[][] board = new int[9][9];
for (int i = 0; i < 9; i++) {
for (int j = 0; j < 9; j++) {
board[i][j] = puzzle[i][j];
}
}
return solveBoard(board, 0, 0);
}
private boolean solveBoard(int[][] board, int row, int col) {
if (col == 9) {
col = 0;
row++;
if (row == 9) {
return true;
}
}
if (board[row][col] != 0) {
return solveBoard(board, row, col + 1);
}
for (int num = 1; num <= 9; num++) {
if (isValid(board, row, col, num)) {
board[row][col] = num;
if (solveBoard(board, row, col + 1)) {
return true;
}
board[row][col] = 0;
}
}
return false;
}
private boolean isValid(int[][] board, int row, int col, int num) {
for (int i = 0; i < 9; i++) {
if (board[row][i] == num || board[i][col] == num) {
return false;
}
}
int boxRow = row - row % 3;
int boxCol = col - col % 3;
for (int i = boxRow; i < boxRow + 3; i++) {
for (int j = boxCol; j < boxCol + 3; j++) {
if (board[i][j] == num) {
return false;
}
}
}
return true;
}
private boolean checkPuzzle() {
int[][] board = new int[9][9];
for (int i = 0; i < 9; i++) {
for (int j = 0; j < 9; j++) {
try {
board[i][j] = Integer.parseInt(cells[i][j].getText());
} catch (NumberFormatException e) {
return false;
}
}
}
for (int i = 0; i < 9; i++) {
for (int j = 0; j < 9; j++) {
if (board[i][j] != solution[i][j]) {
return false;
}
}
}
return true;
}
private void savePuzzle() {
try {
PrintWriter writer = new PrintWriter(new FileWriter("sudoku.txt"));
writer.println("Puzzle:");
for (int i = 0; i < 9; i++) {
for (int j = 0; j < 9; j++) {
writer.print(puzzle[i][j]);
}
writer.println();
}
writer.println("Solution:");
for (int i = 0; i < 9; i++) {
for (int j = 0; j < 9; j++) {
writer.print(solution[i][j]);
}
writer.println();
}
writer.close();
} catch (IOException e) {
JOptionPane.showMessageDialog(this, "Error saving puzzle", "Error", JOptionPane.ERROR_MESSAGE);
}
}
private void loadPuzzle() {
try {
BufferedReader reader = new BufferedReader(new FileReader("sudoku.txt"));
String line;
for (int i = 0; i < 9; i++) {
line = reader.readLine();
for (int j = 0; j < 9; j++) {
puzzle[i][j] = line.charAt(j) - '0';
}
}
line = reader.readLine();
for (int i = 0; i < 9; i++) {
line = reader.readLine();
for (int j = 0; j < 9; j++) {
solution[i][j] = line.charAt(j) - '0';
}
}
reader.close();
updateCells();
} catch (IOException e) {
JOptionPane.showMessageDialog(this, "Error loading puzzle", "Error", JOptionPane.ERROR_MESSAGE);
}
}
private void updateCells() {
for (int i = 0; i < 9; i++) {
for (int j = 0; j < 9; j++) {
if (puzzle[i][j] == 0) {
cells[i][j].setText("");
} else {
cells[i][j].setText(Integer.toString(puzzle[i][j]));
}
}
}
}
private class NewGameListener implements ActionListener {
public void actionPerformed(ActionEvent e) {
if (generatePuzzle()) {
updateCells();
} else {
JOptionPane.showMessageDialog(SudokuGame.this, "Error generating puzzle", "Error", JOptionPane.ERROR_MESSAGE);
}
}
}
private class CheckListener implements ActionListener {
public void actionPerformed(ActionEvent e) {
if (checkPuzzle()) {
JOptionPane.showMessageDialog(SudokuGame.this, "Congratulations! You solved the puzzle!", "Congratulations", JOptionPane.PLAIN_MESSAGE);
} else {
JOptionPane.showMessageDialog(SudokuGame.this, "Sorry, your solution is incorrect.", "Incorrect Solution", JOptionPane.ERROR_MESSAGE);
}
}
}
private class SaveListener implements ActionListener {
public void actionPerformed(ActionEvent e) {
savePuzzle();
}
}
private class LoadListener implements ActionListener {
public void actionPerformed(ActionEvent e) {
loadPuzzle();
}
}
public static void main(String[] args) {
SudokuGame game = new SudokuGame();
game.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
```
这个程序实现了数独游戏的基本功能,包括产生指定形式的数独题目、在产生的数独题目上,程序产生数独的答案、将题目和答案保存在文件、用户可按指定格式输入数独答案、程序可以检查答案是否正确。你可以根据自己的需要对程序进行修改和扩展。
用Java实现数独游戏;1、要求产生指定形式的数独题目 2、在产生的数独题目上,程序产生数独的答案,将题目和答案保存在文件。 3、用户可按指定格式输入数独答案,程序可以检查答案是否正确,如果不正确,给出参考答案。
好的,这是一个比较复杂的项目,需要多个步骤来实现。以下是我建议的步骤:
1. 生成数独题目
生成数独题目的方法有很多种,这里我介绍一种比较简单的方法。首先,我们需要一个数独的空白模板,即一个二维数组,数组中的每个元素都是一个数字,表示这个位置上应该填什么数。比如下面这个空白模板:
```
int[][] template = {
{0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0}
};
```
其中,0 表示这个位置上还没有填数字。接下来,我们需要填充这个模板,生成一个完整的数独题目。填充的方法如下:
- 先随机选取一行,将这一行中空白位置的数字填上 1-9 中的一个。
- 检查这一行是否合法,即这一行中没有重复的数字。
- 如果这一行合法,则继续填下一行,否则重新填这一行。
- 当所有行都填满数字时,得到一个完整的数独题目。
代码实现如下:
```
private static void generateSudoku(int[][] template) {
Random random = new Random();
for (int i = 0; i < 9; i++) {
int[] row = template[i];
List<Integer> candidates = new ArrayList<>();
for (int j = 1; j <= 9; j++) {
candidates.add(j);
}
for (int j = 0; j < 9; j++) {
if (row[j] != 0) {
candidates.remove(Integer.valueOf(row[j]));
}
}
while (candidates.size() > 0) {
int index = random.nextInt(candidates.size());
int candidate = candidates.get(index);
row = Arrays.copyOf(row, row.length);
row[findEmptyIndex(row)] = candidate;
if (isValidRow(row)) {
template[i] = row;
break;
} else {
candidates.remove(index);
}
}
}
}
private static int findEmptyIndex(int[] row) {
for (int i = 0; i < row.length; i++) {
if (row[i] == 0) {
return i;
}
}
return -1;
}
private static boolean isValidRow(int[] row) {
Set<Integer> set = new HashSet<>();
for (int i = 0; i < row.length; i++) {
if (row[i] == 0) {
continue;
}
if (set.contains(row[i])) {
return false;
} else {
set.add(row[i]);
}
}
return true;
}
```
2. 生成数独答案
生成数独答案的方法也可以用类似的方式,不过需要更加谨慎,保证填好的数字不会影响到后面的填数过程。具体方法可以参考这篇文章:https://www.zhihu.com/question/49157649/answer/115417341
3. 将题目和答案保存在文件
保存题目和答案的方法也比较简单,只需要将二维数组中的数字按照指定格式输出到文件中即可。比如,可以将每行数字用逗号分隔,每个数字之间用空格分隔,空白位置用 0 表示。例如:
```
0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0
1 2 3 4 5 6 7 8 9
4 5 6 7 8 9 1 2 3
7 8 9 1 2 3 4 5 6
2 3 4 5 6 7 8 9 1
5 6 7 8 9 1 2 3 4
8 9 1 2 3 4 5 6 7
3 4 5 6 7 8 9 1 2
6 7 8 9 1 2 3 4 5
9 1 2 3 4 5 6 7 8
```
保存文件的方法可以用 Java 的文件操作 API 实现,不再赘述。
4. 检查答案是否正确
检查答案是否正确的方法比较简单,只需要逐行逐列检查是否有重复的数字即可。代码实现如下:
```
private static boolean checkAnswer(int[][] template, int[][] answer) {
for (int i = 0; i < 9; i++) {
if (!isValidRow(answer[i]) || !isValidColumn(answer, i) || !isValidBlock(answer, i)) {
return false;
}
}
for (int i = 0; i < 9; i++) {
for (int j = 0; j < 9; j++) {
if (template[i][j] != 0 && template[i][j] != answer[i][j]) {
return false;
}
}
}
return true;
}
private static boolean isValidColumn(int[][] answer, int columnIndex) {
Set<Integer> set = new HashSet<>();
for (int i = 0; i < 9; i++) {
int num = answer[i][columnIndex];
if (num == 0) {
continue;
}
if (set.contains(num)) {
return false;
} else {
set.add(num);
}
}
return true;
}
private static boolean isValidBlock(int[][] answer, int blockIndex) {
Set<Integer> set = new HashSet<>();
int rowStart = (blockIndex / 3) * 3;
int colStart = (blockIndex % 3) * 3;
for (int i = rowStart; i < rowStart + 3; i++) {
for (int j = colStart; j < colStart + 3; j++) {
int num = answer[i][j];
if (num == 0) {
continue;
}
if (set.contains(num)) {
return false;
} else {
set.add(num);
}
}
}
return true;
}
```
这样,一个简单的 Java 实现数独游戏的项目就完成了。
阅读全文
相关推荐
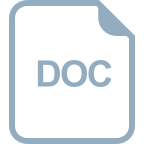
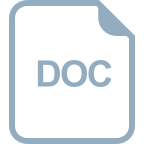
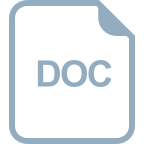
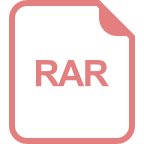
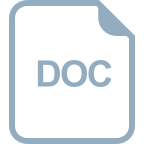
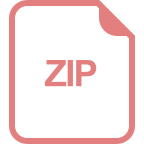
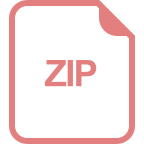
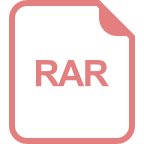
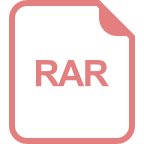
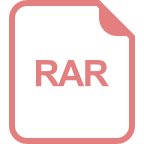
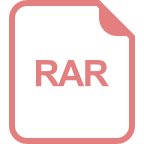
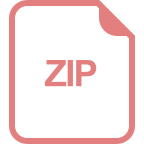
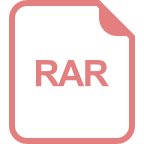
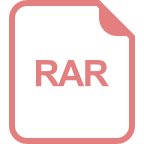
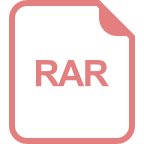
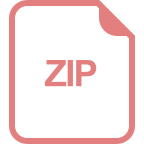
