java通过用户使用协同过滤算法推荐商品代码怎样实现
时间: 2024-05-13 08:21:11 浏览: 156
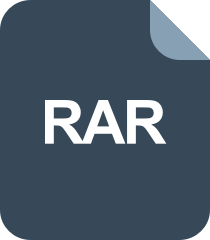
Java项目之基于协同过滤算法商品推荐系统(源码)

以下是一个简单的Java实现协同过滤推荐算法的示例代码:
```
public class UserCF {
private Map<Integer, Map<Integer, Double>> userItemRatingMatrix;
public UserCF(Map<Integer, Map<Integer, Double>> userItemRatingMatrix) {
this.userItemRatingMatrix = userItemRatingMatrix;
}
public Map<Integer, Double> recommendItems(int userId, int neighborNum, int recommendNum) {
// 所有用户的相似度列表
Map<Integer, Double> simMap = calcAllSim(userId);
// 前k个相似用户的id
List<Integer> neigborIds = findTopK(simMap, neighborNum);
// 前k个相似用户对于商品的评分加权平均
Map<Integer, Double> itemScoreMap = new HashMap<>();
for (int neighborId : neigborIds) {
Map<Integer, Double> itemRatingMap = userItemRatingMatrix.get(neighborId);
for (int itemId : itemRatingMap.keySet()) {
if (!userItemRatingMatrix.get(userId).containsKey(itemId)) {
itemScoreMap.merge(itemId, itemRatingMap.get(itemId) * simMap.get(neighborId), Double::sum);
}
}
}
// 推荐前n个商品
List<Map.Entry<Integer, Double>> itemList = new ArrayList<>(itemScoreMap.entrySet());
itemList.sort((o1, o2) -> o2.getValue().compareTo(o1.getValue()));
Map<Integer, Double> recommendMap = new LinkedHashMap<>();
int i = 0;
for (Map.Entry<Integer, Double> entry : itemList) {
recommendMap.put(entry.getKey(), entry.getValue());
i++;
if (i >= recommendNum) {
break;
}
}
return recommendMap;
}
private double calcSim(int userId1, int userId2) {
Map<Integer, Double> ratings1 = userItemRatingMatrix.get(userId1);
Map<Integer, Double> ratings2 = userItemRatingMatrix.get(userId2);
double sumOfSquares1 = 0.0, sumOfSquares2 = 0.0, sumOfProducts = 0.0;
for (int itemId : ratings1.keySet()) {
if (ratings2.containsKey(itemId)) {
double rating1 = ratings1.get(itemId), rating2 = ratings2.get(itemId);
sumOfSquares1 += rating1 * rating1;
sumOfSquares2 += rating2 * rating2;
sumOfProducts += rating1 * rating2;
}
}
if (sumOfProducts == 0.0) {
return 0.0;
} else {
return sumOfProducts / Math.sqrt(sumOfSquares1 * sumOfSquares2);
}
}
private Map<Integer, Double> calcAllSim(int userId) {
Map<Integer, Double> simMap = new HashMap<>();
Map<Integer, Double> ratings = userItemRatingMatrix.get(userId);
for (int neighborId : userItemRatingMatrix.keySet()) {
if (neighborId != userId) {
simMap.put(neighborId, calcSim(userId, neighborId));
}
}
return simMap;
}
private List<Integer> findTopK(Map<Integer, Double> simMap, int k) {
List<Map.Entry<Integer, Double>> simList = new ArrayList<>(simMap.entrySet());
simList.sort((o1, o2) -> o2.getValue().compareTo(o1.getValue()));
List<Integer> topK = new ArrayList<>();
int i = 0;
for (Map.Entry<Integer, Double> entry : simList) {
topK.add(entry.getKey());
i++;
if (i >= k) {
break;
}
}
return topK;
}
}
```
以上代码基于用户对商品的评分矩阵实现,推荐算法主要分为三步:
1. 计算用户之间的相似度
2. 找到和目标用户最相近的k个用户
3. 对这k个用户评价过但目标用户没评价过的商品进行评分加权平均,得到推荐商品列表
阅读全文
相关推荐
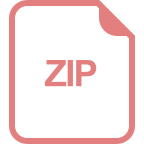
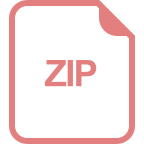
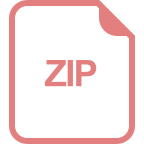
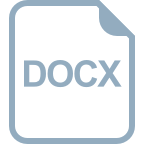




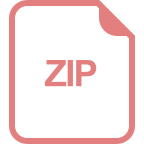
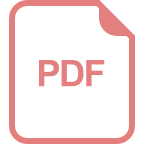
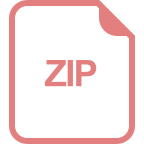
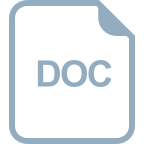
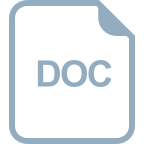
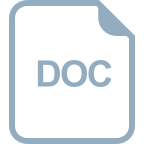
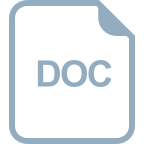