blender python连接两个顶点怎么写
时间: 2023-09-03 17:04:39 浏览: 67
### 回答1:
你可以使用以下代码连接两个选定的顶点:
```python
import bpy
# 选中两个顶点
verts = bpy.context.selected_editable_objects[0].data.vertices
v1 = verts[0]
v2 = verts[1]
# 创建一个新的边
new_edge = bpy.context.selected_editable_objects[0].data.edges.new((v1, v2))
# 使新创建的边成为选中状态
bpy.ops.object.mode_set(mode='OBJECT')
bpy.context.selected_objects = [bpy.context.active_object]
bpy.ops.object.mode_set(mode='EDIT')
bpy.ops.mesh.select_mode(type='EDGE')
bpy.ops.mesh.select_all(action='DESELECT')
new_edge.select_set(True)
```
这个代码段假设你已经选定了两个顶点。它会在这两个顶点之间创建一条新的边,并且选中这条新的边。
### 回答2:
在Blender中,可以使用Python编写脚本来连接两个顶点。下面是一种可能的方法:
1. 首先,你需要导入Blender的Python API模块,可以使用以下代码行来实现:
```python
import bpy
```
2. 确定你要连接的两个顶点的索引。可以使用以下代码来获取所选对象(比如网格对象)的顶点信息和索引:
```python
obj = bpy.context.object
mesh = obj.data
v1_index = 0 # 第一个顶点的索引
v2_index = 1 # 第二个顶点的索引
```
3. 创建一个新的边(连接线)来连接这两个顶点。可以使用以下代码行来创建边:
```python
mesh.edges.add(1) # 添加一个新的边
new_edge = mesh.edges[0] # 获取新创建的边对象
new_edge.vertices[0] = v1_index # 将边的第一个顶点设置为 v1_index
new_edge.vertices[1] = v2_index # 将边的第二个顶点设置为 v2_index
```
4. 更新网格对象的数据,使得边显示在视窗中。可以使用以下代码行来更新网格数据:
```python
mesh.update() # 更新网格数据
```
上述代码示例演示了如何使用Blender的Python API连接两个顶点。你可以根据你的具体需求进行修改和扩展。
### 回答3:
在Blender中,可以使用Python代码连接两个顶点,具体步骤如下:
1. 首先,导入必要的模块:
```python
import bpy
```
2. 创建两个顶点对象:
```python
v1 = bpy.data.objects.new("Vertex1", None)
v2 = bpy.data.objects.new("Vertex2", None)
```
3. 设置两个顶点的坐标:
```python
v1.location = (0, 0, 0) # (x, y, z)
v2.location = (1, 1, 0) # (x, y, z)
```
4. 将两个顶点添加到场景中:
```python
bpy.context.scene.collection.objects.link(v1)
bpy.context.scene.collection.objects.link(v2)
```
5. 创建一条线对象,并将其添加到场景中:
```python
line = bpy.data.objects.new("Line", bpy.data.curves.new("Line", 'CURVE'))
bpy.context.scene.collection.objects.link(line)
```
6. 通过指定起始点和结束点来连接两个顶点:
```python
line.data.splines.new('BEZIER')
line.data.splines[0].bezier_points.add(2)
line.data.splines[0].bezier_points[0].co = v1.location
line.data.splines[0].bezier_points[1].co = v2.location
```
7. (可选)如果需要,可以设置线的样式和属性:
```python
line.data.splines[0].bezier_points[0].handle_right_type = 'VECTOR'
line.data.splines[0].bezier_points[1].handle_left_type = 'VECTOR'
line.data.resolution_u = 2
```
其中,handle_right_type和handle_left_type设置控制点的处理类型,'VECTOR'表示使用向量方式处理。
8. 最后,刷新场景视图以显示连接的线条:
```python
bpy.context.view_layer.update()
```
这样,两个顶点之间就通过一条线连接起来了。根据需要,可以根据上述代码进行修改和扩展。
相关推荐
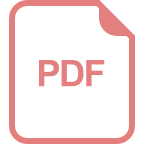
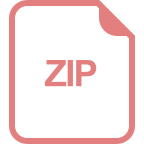
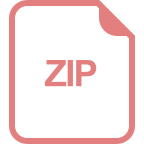














