使用哈夫曼编码实现无损压缩文件
时间: 2024-05-25 19:06:15 浏览: 21
使用哈夫曼编码实现无损压缩文件的步骤如下:
1. 获取原文件中每个字节出现的次数。
2. 根据字节出现的频次信息构建Huffman树。
3. 获取Huffman编码。
4. 使用Huffman编码来改写文件。
具体实现步骤如下:
1. 获取原文件中每个字节出现的次数。可以使用Python中的collections库中的Counter函数来实现。例如:
```python
import collections
with open('original_file.txt', 'rb') as f:
byte_freq = collections.Counter(f.read())
```
2. 根据字节出现的频次信息构建Huffman树。可以使用heapq库中的heappush和heappop函数来实现。例如:
```python
import heapq
def build_huffman_tree(freq):
heap = [[weight, [byte, ""]] for byte, weight in freq.items()]
heapq.heapify(heap)
while len(heap) > 1:
left = heapq.heappop(heap)
right = heapq.heappop(heap)
for pair in left[1:]:
pair[1] = '0' + pair[1]
for pair in right[1:]:
pair[1] = '1' + pair[1]
heapq.heappush(heap, [left[0] + right[0]] + left[1:] + right[1:])
return sorted(heapq.heappop(heap)[1:], key=lambda x: (len(x[-1]), x))
huffman_tree = build_huffman_tree(byte_freq)
```
3. 获取Huffman编码。可以从Huffman树中获取每个字节的编码。例如:
```python
huffman_code = {byte: code for byte, code in huffman_tree}
```
4. 使用Huffman编码来改写文件。可以使用Python中的bitarray库来实现。例如:
```python
import bitarray
with open('original_file.txt', 'rb') as f, open('compressed_file.bin', 'wb') as output:
bit_array = bitarray.bitarray()
bit_array.encode(huffman_code, f.read())
bit_array.tofile(output)
```
相关推荐
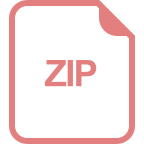
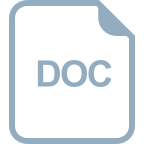
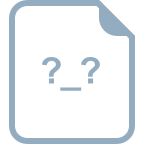
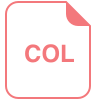
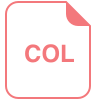
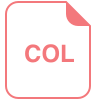
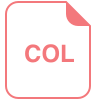
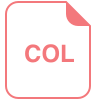









