如何在C++中实现一个函数,以便对浮点数进行四舍五入并保留指定的n位小数?
时间: 2024-10-20 07:03:18 浏览: 38
在C++中,你可以使用`std::fixed`和`std::setprecision`从 `<iomanip>` 头文件来实现一个函数,用于对浮点数进行四舍五入并保留指定的小数位数。下面是一个简单的示例:
```cpp
#include <iostream>
#include <iomanip>
// 定义一个函数 round_to_n_decimal_places
double round_to_n_decimal_places(double number, int n) {
std::ios_base::fmtflags oldFlags = std::cout.flags(); // 保存原始设置
std::cout << std::fixed; // 设置固定小数点精度
std::cout << std::setprecision(n); // 设置保留小数位数
double rounded_number = std::round(number * 10.0^n) / 10.0^n; // 四舍五入
std::cout << rounded_number;
std::cout.flags(oldFlags); // 恢复原来的设置
return rounded_number;
}
int main() {
double input = 3.14159265358979323846;
int precision = 3;
double rounded = round_to_n_decimal_places(input, precision);
std::cout << "Rounded to " << precision << " decimal places: " << rounded << std::endl;
return 0;
}
```
在这个例子中,`round_to_n_decimal_places` 函数接受一个浮点数和一个整数作为参数,然后通过设置`std::setprecision`改变输出的精度,并利用`std::round`函数四舍五入。注意在主函数结束前要恢复原始的`std::ios_base::fmtflags`。
阅读全文
相关推荐
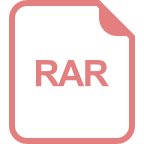
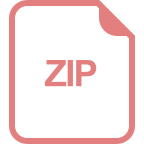
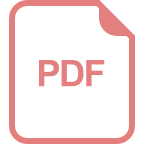















