请帮我实现 VideoFileWriter 它封装了JavaCV库的一些方法,用于创建视频文件、添加帧和音轨等操作
时间: 2024-03-12 17:46:49 浏览: 98
好的,下面是一个简单的VideoFileWriter类的实现,它封装了JavaCV库的一些方法,用于创建视频文件、添加帧和音轨等操作:
```
import java.io.File;
import java.time.Duration;
import org.bytedeco.javacv.FFmpegFrameRecorder;
import org.bytedeco.javacv.Frame;
import org.bytedeco.javacv.Java2DFrameConverter;
import org.bytedeco.javacv.OpenCVFrameConverter;
import org.bytedeco.opencv.global.opencv_imgcodecs;
public class VideoFileWriter {
private final FFmpegFrameRecorder recorder;
private final Java2DFrameConverter imageConverter;
private final OpenCVFrameConverter.ToIplImage opencvConverter;
private boolean isClosed;
private long startTime;
public VideoFileWriter(File outputFile, int width, int height, int fps, int duration) throws Exception {
this.recorder = new FFmpegFrameRecorder(outputFile, width, height);
this.recorder.setFrameRate(fps);
this.recorder.setVideoCodecName("libx264");
this.recorder.setFormat("mp4");
this.recorder.setVideoBitrate(500000);
this.recorder.start();
this.imageConverter = new Java2DFrameConverter();
this.opencvConverter = new OpenCVFrameConverter.ToIplImage();
this.isClosed = false;
this.startTime = System.currentTimeMillis();
}
public void writeFrame(BufferedImage image, Duration duration) throws Exception {
if (isClosed) {
throw new IllegalStateException("VideoFileWriter is closed");
}
Frame frame = imageConverter.convert(image);
recorder.setTimestamp(System.currentTimeMillis() - startTime);
recorder.record(frame);
Thread.sleep(duration.toMillis());
}
public void addAudioTrack(Clip audioClip) throws Exception {
if (isClosed) {
throw new IllegalStateException("VideoFileWriter is closed");
}
AudioInputStream audioStream = audioClip.getAudioInputStream();
AudioFormat audioFormat = audioStream.getFormat();
DataLine.Info info = new DataLine.Info(SourceDataLine.class, audioFormat);
SourceDataLine line = (SourceDataLine) AudioSystem.getLine(info);
line.open(audioFormat);
line.start();
byte[] buffer = new byte[65536];
int bytesRead = 0;
while ((bytesRead = audioStream.read(buffer)) > 0) {
line.write(buffer, 0, bytesRead);
}
line.drain();
line.stop();
line.close();
audioStream.close();
}
public void close() throws Exception {
if (isClosed) {
return;
}
isClosed = true;
recorder.stop();
recorder.release();
}
}
```
在这个类中,我们使用了JavaCV库的FFmpegFrameRecorder、Java2DFrameConverter和OpenCVFrameConverter等类来实现视频文件的创建、帧的添加和音轨的添加等操作。其中,writeFrame方法用于添加帧,addAudioTrack方法用于添加音轨,close方法用于关闭视频文件。
相关推荐
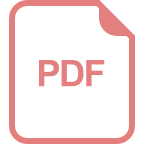
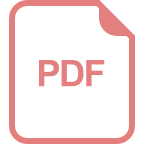
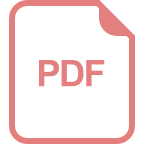
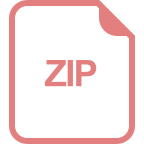
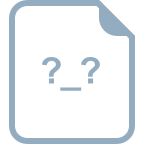
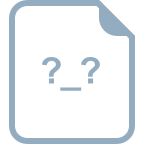
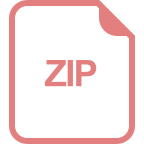
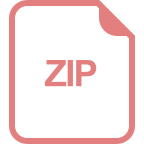
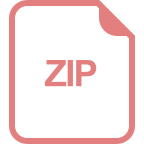
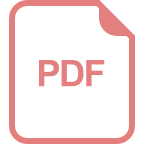
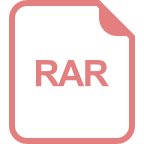
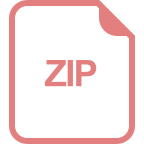
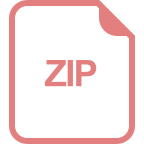
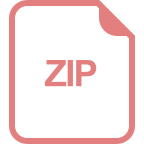
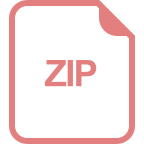
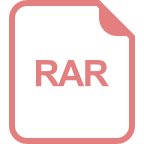
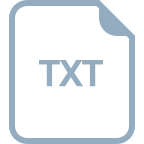