数组存放的完全二叉树中,怎么通过数组加标找到某个结点的左右子树
时间: 2024-02-25 10:59:04 浏览: 20
在一个完全二叉树中,可以通过数组来存储每个节点的信息。假设我们将完全二叉树的根节点存储在数组下标为0的位置,那么对于任意一个下标为i的节点,其左子节点的下标为2i+1,右子节点的下标为2i+2。
因此,如果要找到某个节点的左右子树,可以通过以下方式:
1. 找到该节点在数组中的下标i
2. 左子节点的下标为2i+1,右子节点的下标为2i+2
3. 如果左子节点或右子节点的下标超出了数组的范围,则说明该节点没有对应的子节点
例如,假设我们有一个数组a来存储完全二叉树的节点信息,要找到下标为i的节点的左右子树,可以使用以下代码:
```
int left_child_index = 2*i+1;
int right_child_index = 2*i+2;
if(left_child_index < a.length){
// 左子树存在,可以访问左子树
int left_child = a[left_child_index];
// do something with left_child
}
if(right_child_index < a.length){
// 右子树存在,可以访问右子树
int right_child = a[right_child_index];
// do something with right_child
}
```
注意,上述代码中需要先判断左子节点和右子节点的下标是否超出了数组范围,否则可能会访问到未定义的内存位置,导致程序出错。
相关问题
用一维数组存放完全二叉树
一维数组可以用来存放完全二叉树。完全二叉树是一种特殊的二叉树,它的每个节点都按照从上到下、从左到右的顺序依次排列。我们可以使用数组来表示完全二叉树,其中数组的索引表示节点的位置,数组的值表示节点的值。
具体的存储方式如下:
1. 对于完全二叉树中的第i个节点,它的左子节点的索引为2i,右子节点的索引为2i+1。
2. 对于完全二叉树中的第i个节点,它的父节点的索引为i/2(向下取整)。
下面是一个示例,演示如何使用一维数组存放完全二叉树,并实现先序、中序和后序遍历:
```python
# 定义完全二叉树的一维数组表示
tree = [None, 'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J']
# 先序遍历
def preorder(root):
if root <= len(tree) - 1:
if tree[root] is not None:
print(tree[root], end=' ')
preorder(root * 2) # 遍历左子树
preorder(root * 2 + 1) # 遍历右子树
# 中序遍历
def inorder(root):
if root <= len(tree) - 1:
if tree[root] is not None:
inorder(root * 2) # 遍历左子树
print(tree[root], end=' ')
inorder(root * 2 + 1) # 遍历右子树
# 后序遍历
def postorder(root):
if root <= len(tree) - 1:
if tree[root] is not None:
postorder(root * 2) # 遍历左子树
postorder(root * 2 + 1) # 遍历右子树
print(tree[root], end=' ')
# 演示先序、中序和后序遍历
print("先序遍历结果:")
preorder(1)
print("\n中序遍历结果:")
inorder(1)
print("\n后序遍历结果:")
postorder(1)
```
输出结果:
```
先序遍历结果:
A B D H I E J C F G
中序遍历结果:
H D I B J E A F C G
后序遍历结果:
H I D J E B F G C A
```
存放在数组中的二叉树转换为二叉链表
将存放在数组中的二叉树转换为二叉链表,可以按照先序遍历的顺序逐个将节点添加到链表中。
具体步骤如下:
1. 定义一个指针p,用于遍历数组中的节点。
2. 遍历数组中的节点,对于每一个节点,创建一个新的链表节点,并将节点的值赋给链表节点。将链表节点插入到链表中,插入的位置为p的右子节点。
3. 如果节点有左子节点,递归处理左子节点,并将左子节点的链表头节点作为链表节点的左子节点。
4. 处理完左子节点后,如果节点有右子节点,递归处理右子节点,并将右子节点的链表头节点作为链表节点的右子节点。
5. 处理完右子节点后,返回链表头节点。
下面是一个示例代码,实现了将存放在数组中的二叉树转换为二叉链表:
```python
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
self.left = None
self.right = None
class Solution:
def sortedListToBST(self, head: ListNode) -> TreeNode:
if not head:
return None
# 将链表转换为数组
nodes = []
while head:
nodes.append(head)
head = head.next
# 调用递归函数
return self.buildTree(nodes, 0, len(nodes) - 1)
def buildTree(self, nodes, l, r):
if l > r:
return None
# 取数组中间的节点作为根节点
mid = (l + r) // 2
root = TreeNode(nodes[mid].val)
# 递归处理左子树和右子树
root.left = self.buildTree(nodes, l, mid - 1)
root.right = self.buildTree(nodes, mid + 1, r)
return root
```
注意:这段代码是将链表转换为二叉搜索树的代码,但是思路和将数组中的二叉树转换为二叉链表是类似的。
相关推荐
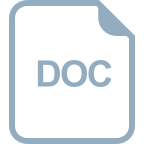
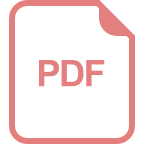
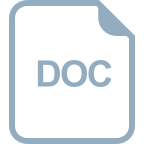












