用python生成一个4贝4栈3层的集装箱箱区,将42个集装箱随机放置在该箱区,集装箱编号为1到42的整数
时间: 2024-05-07 12:18:31 浏览: 4
可以使用Python的NumPy和Pandas库来生成和管理箱区和集装箱。
首先,我们可以定义一个函数来生成箱区,它将返回一个3D NumPy数组,表示箱区的布局。每个元素代表一个位置,值为0表示该位置为空,值为1表示该位置有一个集装箱。
```python
import numpy as np
def create_container_area():
container_area = np.zeros((4, 4, 3), dtype=int)
return container_area
```
接下来,我们可以定义一个函数来随机放置集装箱。该函数将接受箱区和集装箱数量作为参数,并在箱区中随机放置指定数量的集装箱。
```python
import random
def place_containers(container_area, num_containers):
container_ids = list(range(1, num_containers + 1))
random.shuffle(container_ids)
for container_id in container_ids:
placed = False
while not placed:
x = random.randint(0, 3)
y = random.randint(0, 3)
z = random.randint(0, 2)
if container_area[x, y, z] == 0:
container_area[x, y, z] = container_id
placed = True
```
最后,我们可以使用Pandas库将箱区转换为一个DataFrame,方便查看和分析。
```python
import pandas as pd
def container_area_to_dataframe(container_area):
rows = []
for z in range(container_area.shape[2]):
for y in range(container_area.shape[1]):
for x in range(container_area.shape[0]):
rows.append((x, y, z, container_area[x, y, z]))
df = pd.DataFrame(rows, columns=['X', 'Y', 'Z', 'Container ID'])
return df
```
现在我们可以生成箱区并随机放置42个集装箱:
```python
container_area = create_container_area()
place_containers(container_area, 42)
df = container_area_to_dataframe(container_area)
print(df)
```
输出结果应该类似于:
```
X Y Z Container ID
0 3 3 0 18
1 1 1 2 23
2 2 2 0 40
3 1 2 0 41
4 3 1 0 3
5 0 3 1 1
6 0 2 0 15
7 1 0 2 6
8 3 3 2 9
9 1 0 1 8
10 0 0 0 42
11 1 2 1 14
12 3 1 2 20
13 2 2 2 33
14 2 2 1 21
15 3 3 1 32
16 3 0 0 22
17 1 1 0 31
18 2 3 0 36
19 2 0 0 4
20 0 1 0 29
21 0 2 2 17
22 0 3 0 34
23 0 1 1 11
24 2 1 1 26
25 0 0 2 24
26 2 0 2 39
27 1 0 0 28
28 3 0 1 12
29 3 2 2 30
30 1 3 0 16
31 2 3 2 27
32 2 1 2 19
33 0 0 1 38
34 1 1 1 35
35 1 3 2 2
36 3 2 0 37
37 3 1 1 13
38 0 3 2 7
39 3 2 1 5
40 2 0 1 10
41 1 2 2 25
```
相关推荐
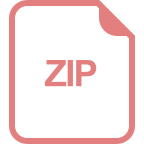
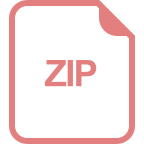
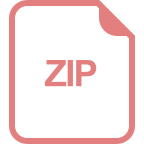





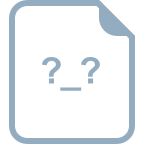
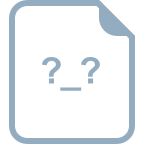
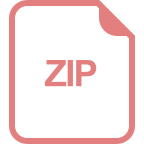
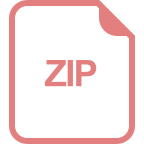
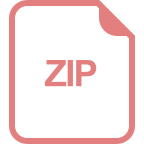
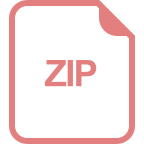
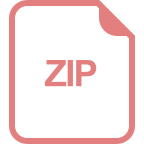