利用回溯法求解最优装载问题
时间: 2024-12-26 09:24:51 浏览: 12
### 使用回溯法实现最优装载问题
#### 问题定义
最优装载问题是关于如何合理分配一组集装箱到两艘具有不同载重量的轮船上,使得所有集装箱都能被装载,并且满足每艘船的最大承载能力。具体来说,给定n个集装箱以及它们各自的重量\( w_i \),还有两艘分别能承受最大重量为 \( c_1 \) 和 \( c_2 \) 的轮船,在总重量不超过两者之和的前提下,尝试找到一种方法来安排这些货物。
#### 解决方案概述
为了利用回溯法解决此问题,可以构建一棵决策树,其中每个节点代表一次选择——即决定某个特定编号的箱子是否放入第一艘船内。当遍历这棵树时,遵循深度优先的原则探索可能的选择路径;一旦发现当前部分解决方案已经违反了任何一艘船只的容量约束,则立即停止对该分支进一步深入考察并且回退至上一层级重新考虑其他可能性直到获得最终解答为止[^4]。
#### 实现细节
以下是基于Python编写的简单版本程序用于演示上述过程:
```python
def backtrack(index, current_weight_ship1, remaining_capacity_ship2):
global best_solution
# 如果所有的物品都已经处理完毕 或者 当前组合超过了第一个货舱的最大承重
if index >= n or current_weight_ship1 > capacity_ship1:
return
# 尝试将index位置处的item加入ship1
if (current_weight_ship1 + weights[index]) <= capacity_ship1:
temp = list(assignment)
temp[index] = True
# 更新最佳解
if sum([weights[i] for i in range(n) if assignment[i]]) + \
sum([weights[j] for j in range(n) if not assignment[j]]) == total_weight and\
all(x<=y for x,y in zip((sum(weights[k]*temp[k] for k in range(len(temp))),
sum(weights[l]*(not temp[l]) for l in range(len(temp)))),
(capacity_ship1,capacity_ship2))):
best_solution = tuple(temp)
# 继续递归调用函数去加载下一个item
backtrack(index + 1, current_weight_ship1 + weights[index], remaining_capacity_ship2 - weights[index])
# 不把index位置处的item加入ship1而是留给ship2的情况
temp = list(assignment)
temp[index] = False
backtrack(index + 1, current_weight_ship1, remaining_capacity_ship2)
if __name__ == "__main__":
from random import randint
# 初始化参数
n = 5 # 物品数量
capacity_ship1 = 8 # 船只1的最大承重
capacity_ship2 = 7 # 船只2的最大承重
weights = [randint(1, min(capacity_ship1, capacity_ship2)) for _ in range(n)] # 各自随机生成一些合理的重量值
total_weight = sum(weights)
print(f"Weights of items are {weights}")
# 开始执行算法之前做一些必要的初始化工作
assignment = [False] * n # 记录哪些项目应该放在哪条船上,默认全部放第二条船上
best_solution = None
# 执行backtrack()函数来进行搜索操作
backtrack(0, 0, capacity_ship2)
if best_solution is not None:
assigned_to_first_ship = [i+1 for i,x in enumerate(best_solution) if x==True]
print(f"The optimal solution assigns these item indices to ship one:{assigned_to_first_ship}.")
else:
print("No feasible solutions found.")
```
这段代码通过模拟一系列二元选择(放置或不放置某件商品),逐步形成完整的分配计划。每当遇到可行的新配置时都会更新全局变量`best_solution`保存下来作为潜在的答案之一。最后输出的是能够成功完成任务的最佳方式下的索引列表。
阅读全文
相关推荐
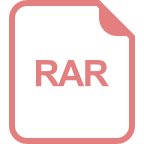
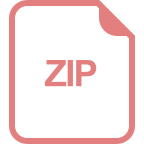



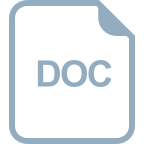
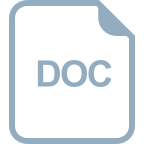


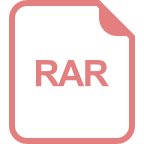
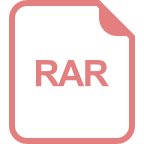




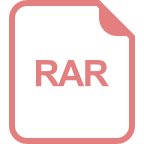
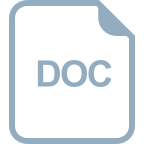
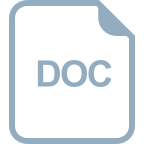