装载问题java_回溯法最优装载问题(java)
时间: 2023-08-05 21:45:39 浏览: 52
最优装载问题是经典的背包问题,可以使用回溯法求解。具体步骤如下:
1. 定义一个数组记录货物的重量和当前已装载的重量;
2. 定义一个变量记录当前最优解;
3. 定义一个回溯函数backtrack,用来递归搜索所有可能的装载方案;
4. 在回溯函数中,遍历所有货物,如果该货物未被装载且装载该货物不会超过最大载重,则装载该货物并更新当前已装载重量;
5. 如果当前已装载重量大于当前最优解,则更新当前最优解;
6. 回溯函数返回后,将当前货物的状态恢复为未装载状态,以便搜索下一个方案。
下面是Java代码实现:
```java
public class OptimalLoad {
private int[] goods; // 货物重量数组
private int maxLoad; // 最大载重
private int curLoad; // 当前已装载重量
private int bestLoad; // 当前最优解
public OptimalLoad(int[] goods, int maxLoad) {
this.goods = goods;
this.maxLoad = maxLoad;
this.curLoad = 0;
this.bestLoad = 0;
}
public int backtrack(int start) {
if (start == goods.length) {
if (curLoad > bestLoad) {
bestLoad = curLoad;
}
return bestLoad;
}
if (curLoad + goods[start] <= maxLoad) {
curLoad += goods[start];
backtrack(start + 1);
curLoad -= goods[start];
}
backtrack(start + 1);
return bestLoad;
}
public static void main(String[] args) {
int[] goods = {2, 2, 4, 6, 3};
int maxLoad = 9;
OptimalLoad optimalLoad = new OptimalLoad(goods, maxLoad);
int bestLoad = optimalLoad.backtrack(0);
System.out.println(bestLoad);
}
}
```
这里给出一个例子:有5个货物,重量分别为2, 2, 4, 6, 3,最大载重为9,求最优装载方案。运行上述代码,会输出最优装载重量为9。
相关推荐









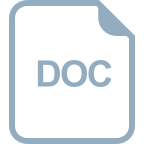
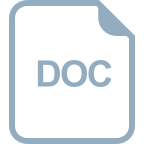
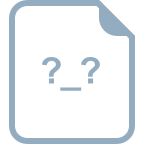