unity字典根据键查找索引
时间: 2023-07-05 17:13:06 浏览: 38
在Unity中,可以使用Dictionary类来实现根据键查找索引的功能。以下是一个简单的示例代码:
```csharp
Dictionary<string, int> myDictionary = new Dictionary<string, int>();
// 添加元素
myDictionary.Add("apple", 0);
myDictionary.Add("banana", 1);
myDictionary.Add("orange", 2);
// 根据键查找索引
int index;
if (myDictionary.TryGetValue("banana", out index))
{
Debug.Log("The index of banana is " + index);
}
else
{
Debug.Log("Banana not found in the dictionary.");
}
```
在上面的代码中,首先创建了一个Dictionary实例,并添加了3个元素。然后使用TryGetValue方法根据键查找索引,如果找到了,则输出索引值;如果没有找到,则输出提示信息。
相关问题
unity 根据索引查找字典元素
在 Unity 中,可以使用以下代码根据索引查找字典元素:
```csharp
// 假设有一个名为 dict 的 Dictionary<int, string>
int index = 0; // 要查找的索引
string value;
if (dict.TryGetValue(index, out value)) {
// 找到了对应索引的元素
Debug.Log("Value at index " + index + ": " + value);
} else {
// 没有找到对应索引的元素
Debug.Log("No value found at index " + index);
}
```
其中,`dict.TryGetValue(index, out value)` 会尝试在字典中查找对应索引的元素,如果找到了就会返回 `true` 并将对应的值赋给 `value` 变量,否则返回 `false`。你可以根据自己的需求进行相应的处理。
unity 字典查找key
在Unity中,你可以使用Dictionary来实现以key-value形式存储和查找数据。要查找Dictionary中的key,可以使用ContainsKey方法来检查key是否存在,如果存在,可以使用索引符号([])或TryGetValue方法来获取对应的value。
示例代码:
```csharp
using System.Collections.Generic;
using UnityEngine;
public class DictionaryExample : MonoBehaviour
{
Dictionary<string, int> scores = new Dictionary<string, int>();
void Start()
{
// 添加数据
scores.Add("Tom", 90);
scores.Add("Jerry", 80);
scores.Add("Alice", 95);
// 查找数据
if (scores.ContainsKey("Tom"))
{
int score = scores["Tom"];
Debug.Log("Tom's score is " + score);
}
int jerryScore;
if (scores.TryGetValue("Jerry", out jerryScore))
{
Debug.Log("Jerry's score is " + jerryScore);
}
}
}
```
相关推荐
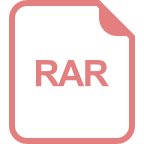
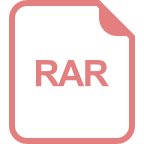




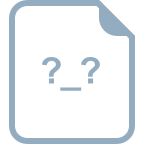
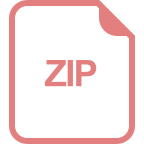
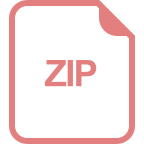
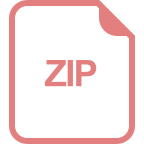
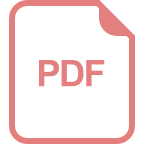
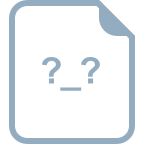
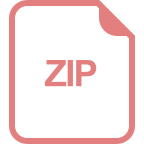