使用c试编写一个求已知单链表的数据域的平均值的函数(数据域数据类型为整型)。
时间: 2024-12-06 19:26:07 浏览: 13
在C语言中,我们可以使用结构体表示链表节点,并编写一个函数来计算链表内所有整数值的平均值。首先,我们需要定义链表节点结构,然后创建一个遍历链表并累加元素值的辅助函数,最后计算平均值。以下是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 链表节点结构
typedef struct ListNode {
int data; // 数据域
struct ListNode* next; // 指向下一个节点的指针
} ListNode;
// 初始化链表节点
ListNode* createNode(int value) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->data = value;
newNode->next = NULL;
return newNode;
}
// 辅助函数遍历链表并累加数据域值
int sumList(ListNode* head) {
int sum = 0;
ListNode* current = head;
while (current != NULL) {
sum += current->data;
current = current->next;
}
return sum;
}
// 计算链表数据域的平均值
double averageListNode(ListNode* head) {
if (head == NULL) { // 如果链表为空,返回0
return 0;
} else {
int sum = sumList(head);
int count = 0; // 统计链表节点数
ListNode* temp = head;
while (temp != NULL) {
count++;
temp = temp->next;
}
return (double)sum / count;
}
}
// 测试案例
int main() {
// 创建链表示例
ListNode* list = createNode(1);
list->next = createNode(2);
list->next->next = createNode(3);
double avg = averageListNode(list);
printf("链表数据域的平均值为: %.2f\n", avg);
return 0;
}
```
在这个示例中,`averageListNode()` 函数接受链表头节点作为输入,通过递归或迭代的方式遍历整个链表,计算所有整数值之和,然后除以节点数得到平均值。
阅读全文
相关推荐
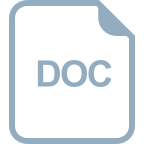
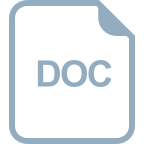
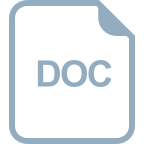




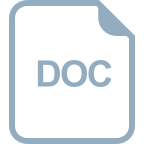
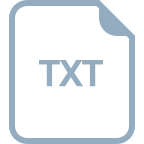
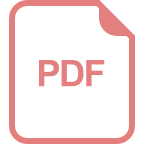
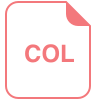
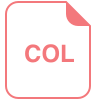
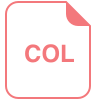
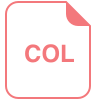
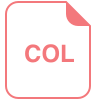
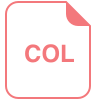
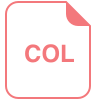
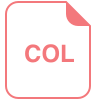