c++ 如何在函数中传递 int 数组
时间: 2024-09-21 16:08:18 浏览: 21
在C++中,你可以通过几种方式在函数中传递整数数组,这里列举了最常见的两种方法:
1. **通过引用(Pass by reference)**:
- 函数接受数组的引用作为参数,可以直接修改原数组内容。
```cpp
void printArray(int arr[], int size) {
for (int i = 0; i < size; i++) {
cout << arr[i] << " ";
}
}
// 调用函数
int myArray[] = {1, 2, 3, 4, 5};
printArray(myArray, sizeof(myArray)/sizeof(myArray[0]));
```
2. **通过指针(Pass by pointer)**:
- 函数接受指向数组首元素的指针,并通过指针访问数组元素。
```cpp
void processArray(int* arr, int size) {
for (int i = 0; i < size; i++) {
arr[i] *= 2; // 双倍数组元素
}
}
// 调用函数
int anotherArray[] = {1, 2, 3, 4, 5};
processArray(anotherArray, sizeof(anotherArray)/sizeof(anotherArray[0]));
for (int i = 0; i < 5; i++) {
cout << anotherArray[i] << " "; // 输出结果为:2 4 6 8 10
}
```
注意,在传递数组时,你需要确保函数接收的数组大小信息正确,或者提供足够的上下文来计算数组大小。另外,为了避免拷贝大量的数据,可以优先考虑使用引用或指针,特别是对于大型数组。
相关推荐
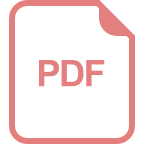
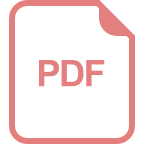
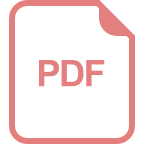














