def channel_shuffle(x, groups): batchsize, num_channels, height, width = x.data.size() channels_per_group = num_channels // groups # reshape x = x.view(batchsize, groups, channels_per_group, height, width) x = torch.transpose(x, 1, 2).contiguous() # flatten x = x.view(batchsize, -1, height, width) return x
时间: 2024-01-16 22:03:30 浏览: 127
这是一个用于通道混洗的函数。输入参数 x 是一个四维的张量,表示一个 batch 内的多张图片;groups 表示要分成的组数。
函数首先从输入张量 x 中获取 batch size、channel 数量、高度和宽度四个维度的大小。然后计算出每组应当包含的 channel 数量,即 channels_per_group = num_channels // groups。
接着,函数将输入张量 x reshape 成新的形状,使得通道数按照 groups 和 channels_per_group 进行划分。具体来说,新的形状为 batchsize * groups * channels_per_group * height * width,其中第二个维度是 groups,第三个维度是 channels_per_group。
接下来,函数使用 torch.transpose() 函数将第二个和第三个维度进行交换,以实现通道混洗的效果。注意,由于交换维度后张量的存储顺序可能不再是连续的,因此需要调用 .contiguous() 方法,使得张量在内存中是连续存储的。
最后,函数将张量再次 reshape,将前两个维度合并为一个,即 batchsize * (groups * channels_per_group) * height * width,并返回结果。
相关问题
import torch import torch.nn as nn class LeNetConvLSTM(nn.Module): def __init__(self, input_size, hidden_size, kernel_size): super(LeNetConvLSTM, self).__init__() # LeNet网络部分 self.conv1 = nn.Conv2d(in_channels=1, out_channels=6, kernel_size=5) self.pool1 = nn.MaxPool2d(kernel_size=2) self.conv2 = nn.Conv2d(in_channels=6, out_channels=16, kernel_size=5) self.pool2 = nn.MaxPool2d(kernel_size=2) self.fc1 = nn.Linear(in_features=16*5*5, out_features=120) self.fc2 = nn.Linear(in_features=120, out_features=84) # ConvLSTM部分 self.lstm = nn.LSTMCell(input_size, hidden_size) self.hidden_size = hidden_size self.kernel_size = kernel_size self.padding = kernel_size // 2 def forward(self, x): # LeNet网络部分 x = self.pool1(torch.relu(self.conv1(x))) x = self.pool2(torch.relu(self.conv2(x))) x = x.view(-1, 16*5*5) x = torch.relu(self.fc1(x)) x = torch.relu(self.fc2(x)) # 将输出转换为ConvLSTM所需的格式 batch_size, channels, height, width = x.shape x = x.view(batch_size, channels, height*width) x = x.permute(0, 2, 1) # ConvLSTM部分 hx = torch.zeros(batch_size, self.hidden_size).to(x.device) cx = torch.zeros(batch_size, self.hidden_size).to(x.device) for i in range(height*width): hx, cx = self.lstm(x[:, i, :], (hx, cx)) hx = hx.view(batch_size, self.hidden_size, 1, 1) cx = cx.view(batch_size, self.hidden_size, 1, 1) if i == 0: output = hx else: output = torch.cat((output, hx), dim=1) # 将输出转换为正常的格式 output = output.permute(0, 2, 3, 1) output = output.view(batch_size, height, width, self.hidden_size) return output
这段代码定义了一个名为 LeNetConvLSTM 的 PyTorch 模型,它实现了一个结合了 LeNet 网络和 ConvLSTM 的神经网络。LeNet 网络用于提取输入数据的特征,ConvLSTM 用于处理时间序列数据。在 forward 方法中,该模型首先通过 LeNet 网络处理输入数据,然后将输出转换为 ConvLSTM 所需的格式,并对每个时间步运行一个 LSTM 单元,最后将 LSTM 单元的输出转换为正常的格式。
class Channel_Att(nn.Module): def __init__(self, channels, t=16): super(Channel_Att, self).__init__() self.channels = channels self.bn2 = nn.BatchNorm2d(self.channels, affine=True) def forward(self, x): residual = x x = self.bn2(x) weight_bn = self.bn2.weight.data.abs() / torch.sum(self.bn2.weight.data.abs()) x = x.permute(0, 2, 3, 1).contiguous() x = torch.mul(weight_bn, x) x = x.permute(0, 3, 1, 2).contiguous() x = torch.sigmoid(x) * residual # return x
这段代码是一个 PyTorch 模型的定义,它实现了通道注意力机制(Channel Attention)。该模块的输入是一个四维张量 x,其形状为 [batch_size, channels, height, width],其中 channels 是输入特征图的通道数。在该模块中,首先对输入 x 进行了一个批归一化(Batch Normalization)操作,然后计算每个通道的权重,即 weight_bn,通过将权重与输入张量 x 点乘来加强或减弱每个通道的表示能力,最后再将得到的结果与原始输入张量相加并经过 sigmoid 激活函数得到输出。
该模块使用了一个 t 参数,其默认值为 16。该参数的作用是在计算权重时引入一个缩放因子,以控制注意力机制的强度。
阅读全文
相关推荐
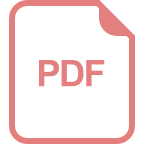

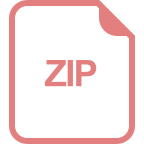
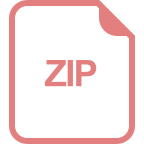
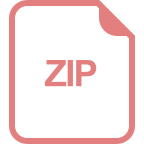
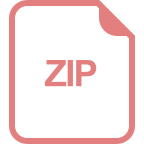
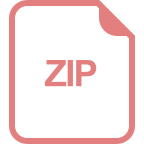
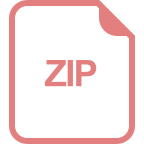
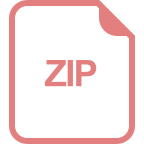
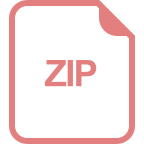
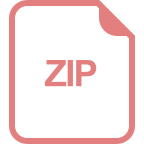
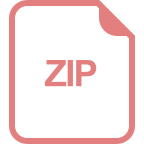

