物理弹球 python 源代码制作
时间: 2023-08-07 09:06:56 浏览: 97
以下是一个使用Python制作物理弹球的示例代码:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 设置窗口尺寸
width, height = 800, 600
screen = pygame.display.set_mode((width, height))
pygame.display.set_caption('弹球游戏')
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
# 定义球的属性
ball_radius = 20
ball_x = random.randint(ball_radius, width - ball_radius)
ball_y = random.randint(ball_radius, height - ball_radius)
ball_speed_x = 5
ball_speed_y = 5
# 定义挡板属性
paddle_width = 100
paddle_height = 20
paddle_x = (width - paddle_width) // 2
paddle_y = height - paddle_height * 2
paddle_speed = 10
# 定义分数和生命值
score = 0
lives = 3
font = pygame.font.Font(None, 30)
# 游戏循环
done = False
clock = pygame.time.Clock()
while not done:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
done = True
# 移动球
ball_x += ball_speed_x
ball_y += ball_speed_y
# 检测碰撞
if ball_x < ball_radius or ball_x > width - ball_radius:
ball_speed_x = -ball_speed_x
if ball_y < ball_radius:
ball_speed_y = -ball_speed_y
elif ball_y > height - ball_radius:
lives -= 1
ball_x = random.randint(ball_radius, width - ball_radius)
ball_y = random.randint(ball_radius, height - ball_radius)
ball_speed_x = 5
ball_speed_y = 5
# 检测挡板碰撞
if ball_y + ball_radius > paddle_y and \
ball_x > paddle_x and \
ball_x < paddle_x + paddle_width:
ball_speed_y = -ball_speed_y
score += 10
# 移动挡板
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT] and paddle_x > 0:
paddle_x -= paddle_speed
if keys[pygame.K_RIGHT] and paddle_x < width - paddle_width:
paddle_x += paddle_speed
# 绘制画面
screen.fill(BLACK)
pygame.draw.circle(screen, RED, (ball_x, ball_y), ball_radius)
pygame.draw.rect(screen, WHITE, (paddle_x, paddle_y, paddle_width, paddle_height))
score_text = font.render('Score: ' + str(score), True, WHITE)
screen.blit(score_text, (10, 10))
lives_text = font.render('Lives: ' + str(lives), True, WHITE)
screen.blit(lives_text, (width - 100, 10))
pygame.display.update()
# 控制帧率
clock.tick(60)
# 退出Pygame
pygame.quit()
```
这个示例使用Pygame库来绘制游戏画面和处理游戏事件。其中,球的属性包括位置和速度,挡板的属性包括位置和速度。游戏循环中,首先处理事件,然后移动球和挡板,检测碰撞,更新分数和生命值,绘制画面并控制帧率。
阅读全文
相关推荐
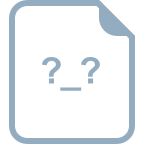
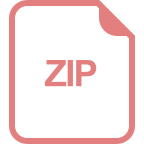
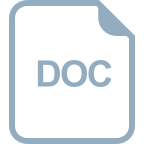
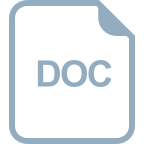
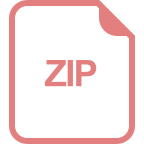
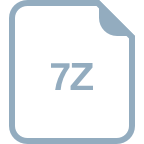
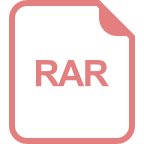
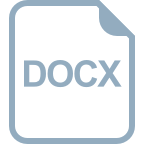
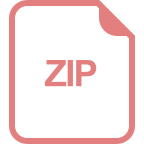
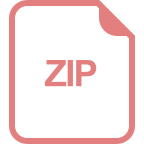
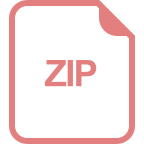
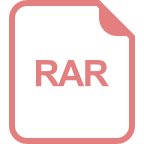
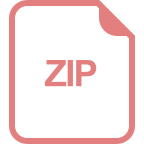
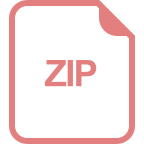
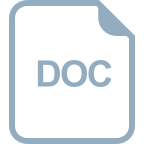
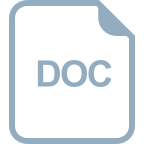
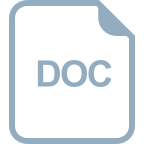