python 多进程间修改同一个文件加锁
时间: 2024-01-06 11:06:45 浏览: 35
在 Python 中,多进程间修改同一个文件时,可以使用文件锁来确保对文件的访问安全。具体来说,可以使用 `fcntl` 模块中的 `flock()` 函数来对文件加锁。在对文件进行写操作时,需要获取文件锁,以确保多个进程之间对文件的访问安全。
下面是一个示例,演示了如何在 Python 中使用文件锁来实现多进程间修改同一个文件:
```python
import os
import fcntl
import time
import multiprocessing
# 定义一个函数,用于修改文件内容
def modify_file(filename):
with open(filename, 'a') as f:
# 获取文件锁
fcntl.flock(f, fcntl.LOCK_EX)
for i in range(10):
f.write("Process {}: {}\n".format(multiprocessing.current_process().name, i))
time.sleep(0.1)
# 释放文件锁
fcntl.flock(f, fcntl.LOCK_UN)
# 创建两个进程并启动它们
process1 = multiprocessing.Process(target=modify_file, args=('test.txt',))
process2 = multiprocessing.Process(target=modify_file, args=('test.txt',))
process1.start()
process2.start()
# 等待进程完成
process1.join()
process2.join()
```
在上面的示例中,我们定义了一个函数 `modify_file()`,用于向指定的文件中写入内容。在对文件进行写操作时,我们使用 `fcntl.flock()` 函数获取文件锁,并在写入完成后释放文件锁。
需要注意的是,文件锁只能保证同一个进程内对文件的访问安全,对于多进程之间的访问,还需要使用其他进程间通信机制,如共享内存、消息队列、管道、套接字等,来确保数据的正确传递。
相关推荐
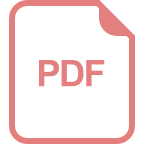














