【Python多线程多进程优化】:pstats模块助你突破性能瓶颈
发布时间: 2024-10-02 05:50:38 阅读量: 3 订阅数: 8 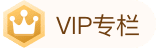
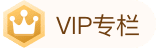
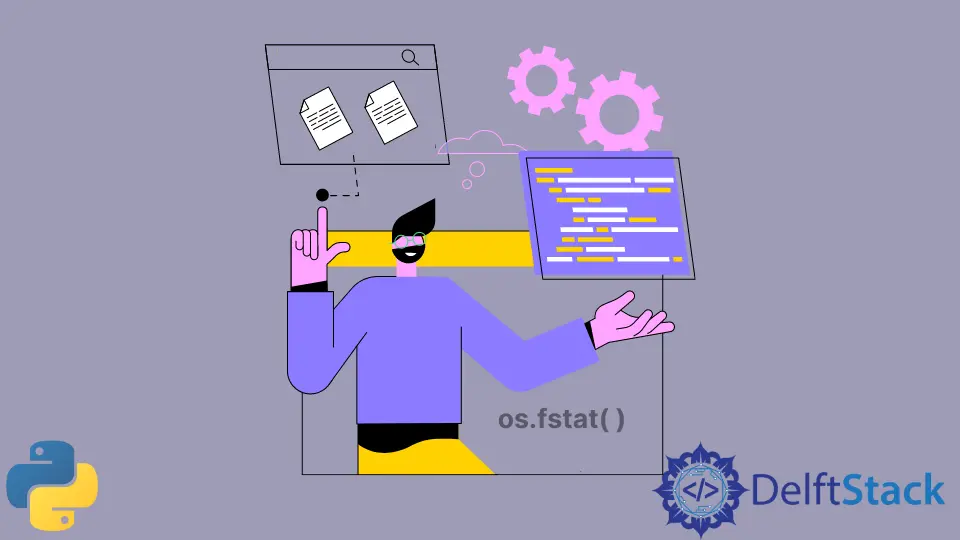
# 1. Python多线程多进程基础
Python是一种广泛使用的高级编程语言,它因简洁的语法和强大的库支持而受到开发者的青睐。在多任务处理方面,Python 提供了两种主要的并发模型:多线程和多进程。本章将介绍这两种并发模型的基础知识,为理解后续章节中的高级概念打下坚实的基础。
## 1.1 多线程编程概述
多线程是一种允许同一程序在多个处理器核心上同时执行多个任务的技术。在Python中,线程由标准库中的`threading`模块提供支持。由于Python的全局解释器锁(GIL)的存在,Python的线程实际上并不能在多核CPU上并行执行Python字节码。然而,它依然适用于I/O密集型任务,在这种情况下,多个线程可以有效地提高程序的响应性和性能。
```python
import threading
import time
def print_numbers():
for i in range(1, 6):
time.sleep(0.1)
print(i)
thread = threading.Thread(target=print_numbers)
thread.start()
thread.join()
```
## 1.2 多进程编程概述
与多线程不同,多进程允许同时运行多个CPU密集型任务,因为每个进程都是一个独立的内存空间,拥有自己的Python解释器和GIL。在Python中,创建和管理进程可以使用`multiprocessing`模块。多进程特别适用于需要进行大量计算或数据处理的任务,每个进程可以利用多核CPU的优势实现真正的并行处理。
```python
import multiprocessing
import time
def task(name):
print(f"Process {name} starting.")
time.sleep(2)
print(f"Process {name} done.")
if __name__ == "__main__":
process_list = []
for i in range(5):
p = multiprocessing.Process(target=task, args=(i,))
process_list.append(p)
p.start()
for process in process_list:
process.join()
```
这两段示例代码分别展示了如何创建一个简单的线程和进程。在后续章节中,我们将深入探讨如何在这些基础之上进一步提升性能,解决多线程和多进程编程中的各种问题。
# 2. Python多线程编程进阶
## 2.1 线程同步机制
### 2.1.1 锁(Lock)的使用
在多线程编程中,确保线程安全的一个重要手段是使用锁。锁确保了在任何给定时间内只有一个线程可以访问某个资源,防止多个线程同时修改资源导致数据不一致的情况。
Python通过`threading`模块提供了`Lock`类,可以用来创建锁对象。以下是使用锁的基本步骤:
```python
import threading
# 创建锁
lock = threading.Lock()
def function():
global balance
lock.acquire() # 尝试获取锁
try:
# 以下操作同一时间只能有一个线程执行
balance += 1
finally:
lock.release() # 释放锁
# 假设创建多个线程执行该函数
threads = []
for i in range(10):
t = threading.Thread(target=function)
threads.append(t)
t.start()
for t in threads:
t.join()
```
在这个例子中,我们有一个全局变量`balance`,多个线程尝试增加这个变量。使用`lock.acquire()`来尝试获取锁,并在操作完成后使用`lock.release()`释放锁。`lock.acquire()`是阻塞操作,如果锁已经被其他线程获取,当前线程将等待直到锁被释放。
使用锁虽然可以解决线程安全问题,但是如果不当使用也会导致死锁等问题,因此需要谨慎设计加锁和解锁的逻辑。
### 2.1.2 信号量(Semaphore)的高级应用
信号量是另一种同步机制,它可以限制对某个资源访问的线程数量,比锁更为灵活。`threading`模块中的`Semaphore`类可以创建一个信号量对象,初始化时指定最多允许多少线程同时访问资源。
```python
import threading
# 创建信号量,最多允许2个线程同时访问
semaphore = threading.Semaphore(2)
def function():
semaphore.acquire() # 尝试获取许可
try:
# 访问资源的代码
pass
finally:
semaphore.release() # 释放许可
# 假设创建多个线程执行该函数
threads = []
for i in range(10):
t = threading.Thread(target=function)
threads.append(t)
t.start()
for t in threads:
t.join()
```
在本例中,我们限制最多有2个线程可以同时访问资源。信号量通常用于限制对共享资源访问的并发数,例如限制数据库连接数、对信号量资源进行计数等场景。
信号量的高级应用还包括二进制信号量,它只允许一个线程通过,用法与普通信号量类似。
## 2.2 线程间通信
### 2.2.1 条件变量(Condition)的原理和使用
条件变量允许线程在某个条件成立之前进入等待状态,并让其他线程在条件成立时通知等待的线程。这是一种线程间同步的方式,可以帮助线程在满足特定条件时才执行特定操作。
在Python中,通过`threading.Condition`来实现条件变量:
```python
import threading
condition = threading.Condition()
def thread1():
with condition:
print("Thread 1: wait for condition")
condition.wait() # 等待条件成立
print("Thread 1: condition met")
def thread2():
with condition:
print("Thread 2: do something")
condition.notify() # 通知等待条件的线程
print("Thread 2: condition notified")
# 创建线程
t1 = threading.Thread(target=thread1)
t2 = threading.Thread(target=thread2)
# 启动线程
t1.start()
t2.start()
```
在这个示例中,`Thread 1`执行`wait`操作后,会进入等待状态直到`Thread 2`执行`notify`。在实际应用中,条件变量常用于实现生产者-消费者模型、协调线程间复杂交互。
### 2.2.2 队列(Que)在多线程中的作用
队列是多线程环境下常用的线程安全的数据结构,用于线程间的任务传递、资源分配等。Python的`queue`模块提供了线程安全的队列类,如`Queue`、`LifoQueue`、`PriorityQueue`等。
使用队列可以避免复杂的线程间同步问题,提高程序的可读性和可维护性。下面是一个使用`Queue`的例子:
```python
import threading
import queue
# 创建一个队列实例
q = queue.Queue()
def producer():
for i in range(5):
item = f'item {i}'
q.put(item) # 生产者将元素放入队列
print(f'Produced {item}')
def consumer():
while True:
item = q.get() # 消费者从队列中获取元素
print(f'Consumed {item}')
q.task_done()
# 创建生产者线程
producer_thread = threading.Thread(target
```
0
0
相关推荐
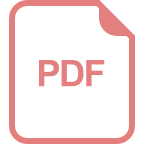
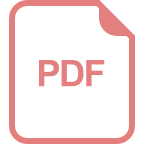
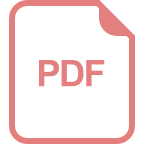






