thinkphp 微信提现代码
时间: 2023-06-14 08:04:49 浏览: 147
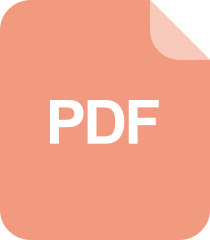
PHP实现微信提现功能
以下是一个简单的ThinkPHP 5.1版本的微信提现代码示例:
```php
<?php
namespace app\index\controller;
use think\Controller;
use think\facade\Config;
use think\facade\Log;
use think\facade\Request;
class Withdraw extends Controller
{
// 微信提现接口
public function wechatWithdraw()
{
// 获取请求参数
$params = Request::param();
// 获取微信支付配置信息
$wxPayConfig = Config::get('wxpay');
// 请求参数构造
$data = [
'mch_appid' => $wxPayConfig['appid'],
'mchid' => $wxPayConfig['mchid'],
'nonce_str' => md5(uniqid()),
'partner_trade_no' => $params['order_no'],
'openid' => $params['openid'],
'check_name' => 'NO_CHECK', // 不校验真实姓名
'amount' => $params['amount'], // 提现金额,单位分
'desc' => '提现描述',
'spbill_create_ip' => $_SERVER['REMOTE_ADDR'],
];
// 生成签名
$data['sign'] = $this->makeSign($data, $wxPayConfig['key']);
// 数组转xml格式
$xmlData = $this->arrayToXml($data);
// 发送请求
$url = 'https://api.mch.weixin.qq.com/mmpaymkttransfers/promotion/transfers';
$response = $this->postXmlCurl($xmlData, $url, true, 6, $wxPayConfig['sslcert_path'], $wxPayConfig['sslkey_path']);
// 解析返回的xml数据
$result = $this->xmlToArray($response);
// 返回结果
if ($result['return_code'] == 'SUCCESS' && $result['result_code'] == 'SUCCESS') {
// 提现成功
return json(['code' => 0, 'msg' => '提现成功']);
} else {
// 提现失败
Log::error('微信提现失败:' . $response);
return json(['code' => 1, 'msg' => '提现失败,请重试']);
}
}
// 生成签名
private function makeSign($data, $key)
{
// 去除数组中的空值
$data = array_filter($data);
// 按照键名的字典序排序
ksort($data);
// 生成字符串
$stringA = '';
foreach ($data as $key => $value) {
$stringA .= $key . '=' . $value . '&';
}
$stringA .= 'key=' . $key;
// 进行MD5签名并转化为大写
return strtoupper(md5($stringA));
}
// 数组转xml格式
private function arrayToXml($data)
{
$xml = "<xml>";
foreach ($data as $key => $value) {
if (is_numeric($value)) {
$xml .= "<" . $key . ">" . $value . "</" . $key . ">";
} else {
$xml .= "<" . $key . "><![CDATA[" . $value . "]]></" . $key . ">";
}
}
$xml .= "</xml>";
return $xml;
}
// 发送post请求xml数据
private function postXmlCurl($xmlData, $url, $useCert = false, $timeout = 30, $sslcert_path = '', $sslkey_path = '')
{
$ch = curl_init();
curl_setopt($ch, CURLOPT_TIMEOUT, $timeout);
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, false);
curl_setopt($ch, CURLOPT_HEADER, false);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $xmlData);
if ($useCert == true) {
curl_setopt($ch, CURLOPT_SSLCERTTYPE, 'PEM');
curl_setopt($ch, CURLOPT_SSLCERT, $sslcert_path);
curl_setopt($ch, CURLOPT_SSLKEYTYPE, 'PEM');
curl_setopt($ch, CURLOPT_SSLKEY, $sslkey_path);
}
$data = curl_exec($ch);
curl_close($ch);
return $data;
}
// xml转数组
private function xmlToArray($xml)
{
// 禁止引用外部xml实体
libxml_disable_entity_loader(true);
$xmlString = simplexml_load_string($xml, 'SimpleXMLElement', LIBXML_NOCDATA);
$result = json_decode(json_encode($xmlString), true);
return $result;
}
}
```
其中,$wxPayConfig是微信支付的配置信息,可以在config目录下的wxpay.php文件中进行配置。
该示例仅供参考,实际使用时需要根据自己的业务需求进行相应的修改和完善。
阅读全文
相关推荐
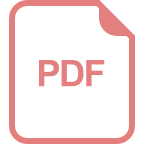
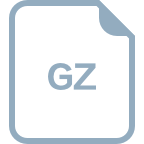















