制作一个jsp查询记录页面
时间: 2024-02-06 17:12:43 浏览: 24
好的,以下是制作一个JSP查询记录页面的简单步骤:
1. 创建一个JSP页面,命名为"search.jsp",并添加一个表单和查询按钮,如下所示:
```
<!DOCTYPE html>
<html>
<head>
<title>Search Records</title>
</head>
<body>
<h1>Search Records</h1>
<form method="post" action="searchResult.jsp">
<label for="name">Name:</label>
<input type="text" id="name" name="name">
<input type="submit" value="Search">
</form>
</body>
</html>
```
2. 创建另一个JSP页面,命名为"searchResult.jsp",用于显示查询结果。在该页面中,获取查询参数并使用JDBC API执行SQL查询,如下所示:
```
<%@ page import="java.sql.*" %>
<!DOCTYPE html>
<html>
<head>
<title>Search Results</title>
</head>
<body>
<h1>Search Results</h1>
<table border="1">
<tr>
<th>Name</th>
<th>Email</th>
<th>Phone</th>
</tr>
<%
String name = request.getParameter("name");
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password");
String sql = "SELECT * FROM records WHERE name LIKE '%" + name + "%'";
pstmt = conn.prepareStatement(sql);
rs = pstmt.executeQuery();
while (rs.next()) {
String recordName = rs.getString("name");
String email = rs.getString("email");
String phone = rs.getString("phone");
out.println("<tr><td>" + recordName + "</td><td>" + email + "</td><td>" + phone + "</td></tr>");
}
} catch (ClassNotFoundException | SQLException e) {
e.printStackTrace();
} finally {
try {
if (rs != null) rs.close();
if (pstmt != null) pstmt.close();
if (conn != null) conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
%>
</table>
</body>
</html>
```
在这个示例中,我们使用了MySQL数据库来存储记录信息。您需要根据实际情况更改数据库连接信息。在JSP页面中,我们使用了JDBC API来执行SQL查询,并将结果呈现为HTML表格。
3. 在Tomcat或其他Web服务器中部署JSP应用程序,并在浏览器中访问"search.jsp"页面进行查询。
希望这个简单的示例对你有所帮助。
相关推荐
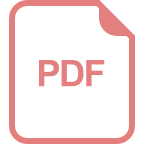
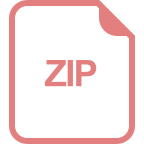
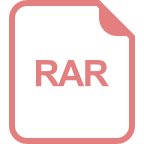
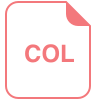













