python read json
时间: 2024-03-21 16:35:58 浏览: 26
在Python中,读取JSON数据可以使用内置的json模块。下面是一个简单的示例代码,演示如何读取JSON文件并将其解析为Python对象:
```python
import json
# 读取JSON文件
with open('data.json', 'r') as file:
data = json.load(file)
# 解析JSON数据
name = data['name']
age = data['age']
city = data['city']
# 打印解析结果
print(f"Name: {name}")
print(f"Age: {age}")
print(f"City: {city}")
```
在上面的代码中,我们首先使用`open()`函数打开JSON文件,并使用`json.load()`函数将文件内容加载为Python对象。然后,我们可以通过键访问JSON对象的值,并将其存储在变量中。最后,我们打印出解析结果。
请注意,上述代码假设JSON文件的内容如下所示:
```json
{
"name": "John",
"age": 25,
"city": "New York"
}
```
相关问题
python读写json
读取JSON文件可以使用json模块的load函数来读取json文件,代码如下:
```python
import json
with open('data.json', 'r') as f:
data = json.load(f)
```
另外,也可以使用json模块的loads函数来读取json字符串,代码如下:
```python
import json
with open('data.json', 'r') as f:
data_str = f.read()
data = json.loads(data_str)
```
写入JSON文件可以使用json模块的dump函数来写入json文件,代码如下:
```python
import json
data = {'name': 'Tom', 'age': 18}
with open('data.json', 'w') as f:
json.dump(data, f)
```
另外,也可以使用json模块的dumps函数将字典转换为json字符串并写入文件,代码如下:
```python
import json
data = {'name': 'Tom', 'age': 18}
with open('data.json', 'w') as f:
data_str = json.dumps(data)
f.write(data_str)
```
以上是使用json模块进行读写json文件的方法。\[1\]\[2\]
#### 引用[.reference_title]
- *1* *2* [python读写json文件方法详解](https://blog.csdn.net/qq_42751978/article/details/130668983)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *3* [Python读写json数据详解](https://blog.csdn.net/qq_37189082/article/details/124654767)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
python导入json
可以使用Python内置的json模块来导入json文件。具体步骤如下:
1. 打开json文件,读取其中的内容。
2. 使用json.loads()方法将读取到的内容转换为Python对象。
以下是示例代码:
```python
import json
# 打开json文件,读取其中的内容
with open('example.json', 'r') as f:
data = f.read()
# 将读取到的内容转换为Python对象
obj = json.loads(data)
```
其中,'example.json'是你要导入的json文件名。
相关推荐
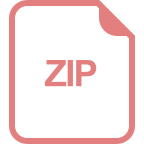
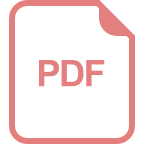
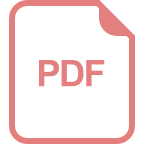












