python在一个字符串列表中,删除以字母a开头的字符串元素
时间: 2024-05-03 11:22:01 浏览: 124
可以使用列表推导式和字符串方法startswith()来实现:
```python
string_list = ['apple', 'banana', 'orange', 'avocado', 'kiwi']
string_list = [s for s in string_list if not s.startswith('a')]
print(string_list)
```
输出:
```
['banana', 'orange', 'kiwi']
```
解释:
`[s for s in string_list if not s.startswith('a')]`是一个列表推导式,它遍历原字符串列表中的每个元素`s`,如果`s`不以字母'a'开头,则将其保留到新列表中。`not s.startswith('a')`是一个布尔表达式,表示如果`s`不以字母'a'开头,则返回True,否则返回False。
相关问题
用Python编写一个函数,接受一个字符串元组和一个字母作为参数,返回该字符串中所有以该字母开头的单词列表
以下是使用Python编写的函数代码:
```python
def get_words_starting_with_letter(words, letter):
result = []
for word in words:
if word.startswith(letter):
result.append(word)
return result
```
这个函数接受两个参数:一个字符串元组 `words` 和一个字母 `letter`。它使用一个循环遍历 `words` 中的每个字符串,并检查它是否以 `letter` 开头。如果是,它将该字符串添加到结果列表 `result` 中。最后,函数返回结果列表。
以下是一个示例:
```python
words = ('apple', 'banana', 'orange', 'apricot', 'melon')
letter = 'a'
result = get_words_starting_with_letter(words, letter)
print(result) # 输出 ['apple', 'apricot']
```
在这个示例中,输入的字符串元组是 `('apple', 'banana', 'orange', 'apricot', 'melon')`,字母是 `'a'`。函数将返回一个列表 `['apple', 'apricot']`,因为它们是以 `'a'` 开头的字符串。
python用户输入一个字符串,请将字符串中的所有字母全部向后移动一位,最后一个字母放到字符串开头,最后将新字符串输出。
好的,我可以回答这个问题。以下是Python代码实现该功能:
```
# 获取用户输入的字符串
s = input("请输入一个字符串:")
# 将字符串中的每个字符向后移动一位
new_s = ''
for c in s:
# 判断字符是否为最后一个字符
if c == s[-1]:
new_s += s[0]
else:
new_s += chr(ord(c) + 1)
# 输出新字符串
print("移动后的字符串为:", new_s)
```
希望能够帮到你。
阅读全文
相关推荐
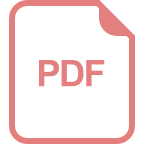













