typedef struct tagDEFINITION_OF_RECORD_step1 { int isEnd; const char *cSearchItem; const char *cDisplayItem; const char *cWitchSettingItem; const char *cSettingItem; } UVS_STATICDB_RECORD_step1; typedef struct tagDEFINITION_OF_SETTING_TABLE_step1 { UVS_STATICDB_RECORD_step1 record[UVS_STATICDB_SETTING_RECORD_NUM_MAX]; } UVS_STATICDB_SETTING_TABLE_step1; typedef struct tagDEFINITION_OF_DB_step1 { const char *language; UVS_STATICDB_SETTING_TABLE_step1 tblSetting; } UVS_STATICDB_step1; extern UVS_STATICDB_step1 g_static_database_step1[]; 怎么给g_static_database_step1循环赋值 g_static_database_step1多少条数据不固定
时间: 2023-08-02 15:06:58 浏览: 39
根据上面提供的代码,`g_static_database_step1`是一个数组,其元素类型为`UVS_STATICDB_step1`。因为`UVS_STATICDB_step1`结构体中包含一个`UVS_STATICDB_SETTING_TABLE_step1`类型的成员变量`tblSetting`,而`UVS_STATICDB_SETTING_TABLE_step1`结构体中包含一个`UVS_STATICDB_RECORD_step1`类型的数组`record`,因此,需要对这个多重结构体进行循环赋值。
下面是一个示例代码,它通过循环嵌套对`g_static_database_step1`进行赋值:
```c
// 定义一个包含所有数据的数组
UVS_STATICDB_step1 data[] = {
{
"language1",
{
{
{0, "search1", "display1", "witch1", "setting1"},
{0, "search2", "display2", "witch2", "setting2"},
// ...
}
}
},
{
"language2",
{
{
{0, "search1", "display1", "witch1", "setting1"},
{0, "search2", "display2", "witch2", "setting2"},
// ...
}
}
},
// ...
};
// 计算数组元素个数
int data_len = sizeof(data) / sizeof(UVS_STATICDB_step1);
// 将数据复制到g_static_database_step1数组中
for (int i = 0; i < data_len; i++) {
g_static_database_step1[i] = data[i];
}
```
需要注意的是,`g_static_database_step1`的大小不固定,取决于实际需要存储的数据量。因此,需要在程序中动态分配内存,并确保不会越界。
相关推荐
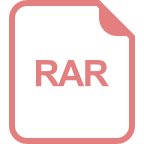
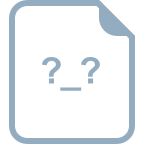
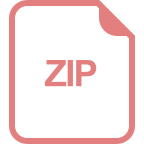
















