okhttp 发起post请求
时间: 2023-09-11 16:13:12 浏览: 77
Android中使用OkHttp进行POST请求的步骤如下:
1. 创建OkHttpClient对象
OkHttpClient client = new OkHttpClient();
2. 创建RequestBody对象
RequestBody requestBody = new FormBody.Builder()
.add("username", "admin")
.add("password", "123456")
.build();
3. 创建Request对象
Request request = new Request.Builder()
.url("http://www.example.com/login")
.post(requestBody)
.build();
4. 发送请求并获取响应
Response response = client.newCall(request).execute();
String result = response.body().string();
其中,第2步中的FormBody.Builder()可以根据实际情况选择其他类型的RequestBody,如Json格式的RequestBody。第3步中的url()方法需要替换成实际的请求地址。第4步中的execute()方法会阻塞当前线程,可以使用enqueue()方法进行异步请求。
相关问题
okhttp3发起post请求
要使用OkHttp3发起POST请求,你可以按照以下步骤进行操作:
1. 首先,你需要创建一个RequestBody对象来包含你要发送的数据。如果你要发送JSON数据,你可以使用RequestBody.create()方法,并将媒体类型设置为"application/json",将JSON数据作为参数传递给该方法。例如:
2. 接下来,你需要创建一个Request对象。通过Request.Builder()方法创建一个Request.Builder对象,并使用url()方法设置请求的URL。然后,使用post()方法将RequestBody对象传递给Request.Builder对象。最后,使用build()方法构建Request对象。例如:
3. 最后,你可以使用OkHttpClient对象来执行请求。通过调用newCall()方法并传递Request对象,创建一个Call对象。然后,使用execute()方法执行Call对象来发送请求,并获取Response对象。你可以通过Response对象来获取响应的状态码和响应数据。例如:
这就是使用OkHttp3发起POST请求的基本步骤。你可以根据你的具体需求进行适当的调整和扩展。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
okhttp3 网络请求
OkHttp3 是一个开源的、高效的网络请求库,主要用于 Android 平台上的网络请求。它支持 HTTP/2、SPDY 和 WebSocket 协议,同时也支持同步和异步请求。下面是一个使用 OkHttp3 发起网络请求的简单示例:
1. 添加依赖
在项目的 build.gradle 文件中添加以下依赖:
```java
implementation 'com.squareup.okhttp3:okhttp:3.14.9'
```
2. 发起 GET 请求
```java
OkHttpClient client = new OkHttpClient();
String url = "https://www.example.com/get";
Request request = new Request.Builder()
.url(url)
.build();
try (Response response = client.newCall(request).execute()) {
if (!response.isSuccessful()) throw new IOException("Unexpected code " + response);
String responseBody = response.body().string();
System.out.println(responseBody);
}
```
3. 发起 POST 请求
```java
OkHttpClient client = new OkHttpClient();
String url = "https://www.example.com/post";
RequestBody requestBody = new FormBody.Builder()
.add("username", "test")
.add("password", "123456")
.build();
Request request = new Request.Builder()
.url(url)
.post(requestBody)
.build();
try (Response response = client.newCall(request).execute()) {
if (!response.isSuccessful()) throw new IOException("Unexpected code " + response);
String responseBody = response.body().string();
System.out.println(responseBody);
}
```
以上就是 OkHttp3 发起网络请求的简单示例,可以根据自己的实际需求进行修改和扩展。
相关推荐
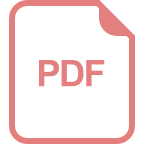
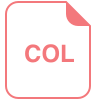
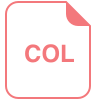
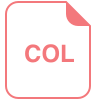
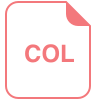
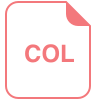







