void fresh_all_light(void){ int i; static char all_slot_color_cmp[50] = {1,2,3,4,5,0,0,0,0,0, 0,0,0,0,0,0,0,0,0,0, 0,0,0,0,0,0,0,0,0,0, 0,0,0,0,0,0,0,0,0,0, 0,0,0,0,0,0,0,0,0,0}; if(memcmp(all_slot_color_cmp,all_slot_color,50) != 0){ fresh_all_io(); memcpy(all_slot_color_cmp,all_slot_color,50); } }
时间: 2023-09-30 17:08:49 浏览: 44
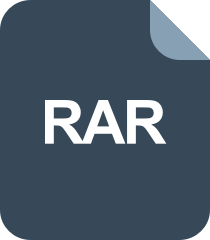
EJB2.0_CMP.rar_cmp_ejb_ejb2 c++
这段代码是一个函数 `fresh_all_light`,它的作用是刷新所有的灯光。让我们逐步分析这段代码:
1. 定义了一个整型变量 `i`,用于循环计数。
2. 定义了一个静态字符数组 `all_slot_color_cmp`,长度为50。数组中的元素用于比较灯光的颜色。
3. 使用了 `memcpy` 函数来将 `all_slot_color` 数组的值复制到 `all_slot_color_cmp` 数组中。
4. 使用了 `memcmp` 函数来比较 `all_slot_color_cmp` 和 `all_slot_color` 两个数组的值是否相等。
5. 如果两个数组的值不相等,则调用 `fresh_all_io` 函数刷新所有的输入/输出。
6. 最后,将 `all_slot_color` 数组的值复制到 `all_slot_color_cmp` 数组中,以便下一次比较。
总体来说,这段代码的作用是检测灯光的颜色是否有变化,如果有变化则刷新输入/输出。
阅读全文
相关推荐
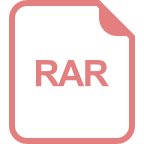
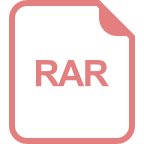
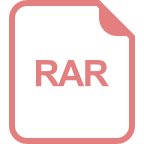
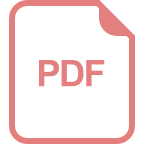
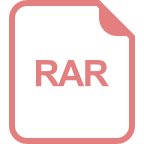
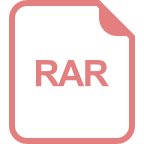
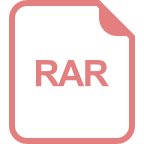








