在python中使用dlib
时间: 2024-03-21 13:19:51 浏览: 28
使用dlib库可以进行人脸检测、人脸对齐、人脸识别等任务。以下是一个简单的使用示例:
1. 安装dlib库
在命令行中执行以下命令:
```
pip install dlib
```
2. 人脸检测
使用dlib库中的`get_frontal_face_detector`函数可以获得一个人脸检测器。然后,将图像作为输入传递给检测器,即可获得检测到的人脸位置。
```python
import dlib
import cv2
# 加载人脸检测器
detector = dlib.get_frontal_face_detector()
# 加载图像
img = cv2.imread('test.jpg')
# 将图像转换为灰度图
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 检测人脸
faces = detector(gray)
# 遍历检测到的人脸,绘制矩形框
for face in faces:
x, y = face.left(), face.top()
w, h = face.right() - x, face.bottom() - y
cv2.rectangle(img, (x, y), (x+w, y+h), (0, 255, 0), 2)
# 显示结果
cv2.imshow("result", img)
cv2.waitKey(0)
```
3. 人脸对齐
使用dlib库中的`get_face_landmarks`函数可以获得人脸68个关键点的坐标。通过这些关键点,可以进行人脸对齐。
```python
import dlib
import cv2
import numpy as np
# 加载人脸检测器和关键点检测器
detector = dlib.get_frontal_face_detector()
predictor = dlib.shape_predictor("shape_predictor_68_face_landmarks.dat")
# 加载图像
img = cv2.imread('test.jpg')
# 将图像转换为灰度图
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 检测人脸
faces = detector(gray)
# 遍历检测到的人脸,进行人脸对齐
for face in faces:
# 获取人脸关键点
landmarks = predictor(gray, face)
# 将关键点转换为numpy数组
landmarks = np.array([(p.x, p.y) for p in landmarks.parts()])
# 计算人脸中心位置
center = landmarks.mean(axis=0).astype("int")
# 计算人脸旋转角度
dy = landmarks[30, 1] - landmarks[27, 1]
dx = landmarks[30, 0] - landmarks[27, 0]
angle = np.degrees(np.arctan2(dy, dx)) - 90
# 构造旋转矩阵
M = cv2.getRotationMatrix2D(tuple(center), angle, scale=1)
# 进行仿射变换
aligned = cv2.warpAffine(img, M, (img.shape[1], img.shape[0]), flags=cv2.INTER_CUBIC)
# 显示结果
cv2.imshow("aligned", aligned)
cv2.waitKey(0)
```
4. 人脸识别
使用dlib库中的`face_recognition`模块可以进行人脸识别。该模块可以计算人脸特征向量,并通过比较特征向量进行人脸匹配。
```python
import dlib
import cv2
import face_recognition
# 加载图像和人脸特征向量
img1 = cv2.imread('test1.jpg')
img2 = cv2.imread('test2.jpg')
face_encoding1 = face_recognition.face_encodings(img1)[0]
face_encoding2 = face_recognition.face_encodings(img2)[0]
# 计算两张图像中人脸的相似度
distance = face_recognition.face_distance([face_encoding1], face_encoding2)
# 显示结果
print("The distance between the two faces is: ", distance[0])
```
以上是dlib库的一些简单使用示例,具体使用方法可以参考dlib官方文档。
相关推荐
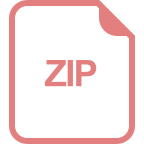
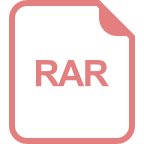














