用c++编写,判断参数x是否满足条件,若是则返回1,若不是则返回0
时间: 2024-02-27 21:58:43 浏览: 41
以下是一个简单的 C++ 函数,用于判断参数 x 是否满足条件,若是则返回 1,若不是则返回 0:
```
int check(int x) {
if (/* 判断条件 */) {
return 1;
} else {
return 0;
}
}
```
你需要自己填写判断条件的部分,使其符合你的要求。
相关问题
用c++编写,判断参数x,x为四位数,x得每位数字的四次方之和与本身相等
以下是一个简单的 C++ 函数,用于判断参数x,x为四位数,x得每位数字的四次方之和与本身相等,如果满足条件,返回1,否则返回0:
```
int check(int x) {
int sum = 0;
int temp = x;
while (temp > 0) {
int digit = temp % 10;
sum += digit * digit * digit * digit;
temp /= 10;
}
if (sum == x) {
return 1;
} else {
return 0;
}
}
```
这个函数会首先计算出参数x的每个数字的四次方之和,然后将其与x本身进行比较,如果相等,就返回1,否则返回0。
平面直线可以用方程表示。按如下要求编写 一个表示平面直线的类 Line。 1)使用私有成员变量表示方程的三个系数。 2)编写构造函数,用于初始化, 三个参数的初始值由函数参数提供。 在构造函数中判断是否满足,如果不满足, 则将 b 赋值为 1。 构造函数参数使用如下默认值 : 。 3)重载构造函数,使用平面点坐标构造直线方程。函数的参数表示两个平面 点 坐 标 , 根 据 这 两 个 坐 标 初 始 化 。 4)编写一个公有成员函数 slope(),返回直线的斜率: 当 ,否则斜率为常数 INFINITY。 5)编写公有成员函数 intercept(), 返回直线的截距: 当 为 ,否则截距为常数 INFINITY。 6) 编 写 一 个 公 有 成 员 函 数 getRelPosTo, 该 函 数 用 于 判 断 直 线 与另一条直线的相对位置关系。判断准则如下: 如果, 两条直线重合(COINCIDENT); 如果, 两条直线平行(PARRALLEL); 其它情况, 两条直线相交(INTERSECT);
抱歉,我可以回答你的问题。以下是 Line 类的实现代码:
```c++
#include <iostream>
#include <cmath>
using namespace std;
class Line {
private:
double a, b, c; // ax + by + c = 0
public:
Line(double aa = 0, double bb = 0, double cc = 0) { // initialize with coefficients
a = aa, b = bb, c = cc;
if (a == 0 && b == 0) b = 1; // if invalid, set b to be 1
}
Line(double x1, double y1, double x2, double y2) { // initialize with two points
if (x1 == x2) {
a = 1, b = 0, c = -x1; // vertical line
} else {
a = (y2 - y1) / (x1 - x2);
b = -1;
c = y1 - a * x1;
}
}
double slope() { // calculate slope
if (b == 0) return INFINITY;
else return -a / b;
}
double intercept() { // calculate intercept
if (b == 0) return INFINITY;
else return -c / b;
}
int getRelPosTo(Line l) { // calculate relative position to another line
if (fabs(a * l.b - b * l.a) < 1e-9 && fabs(b * l.c - c * l.b) < 1e-9)
return COINCIDENT;
if (fabs(a * l.b - b * l.a) < 1e-9) return PARALLEL;
else return INTERSECT;
}
const int COINCIDENT = 0;
const int PARALLEL = 1;
const int INTERSECT = 2;
};
int main() {
Line l1(1, 2, 3); // 1x + 2y + 3 = 0
cout << "Slope: " << l1.slope() << endl; // -0.5
cout << "Intercept: " << l1.intercept() << endl; // -1.5
Line l2(0, 1, 1); // y + 1 = 0, vertical line passing through (1, 0)
cout << "Slope: " << l2.slope() << endl; // INF
cout << "Intercept: " << l2.intercept() << endl; // -1
Line l3(2, 3, 4, 5); // y = x + 1
cout << "Slope: " << l3.slope() << endl; // 1
cout << "Intercept: " << l3.intercept() << endl; // 1
cout << "Relative position to l1: " << l2.getRelPosTo(l1) << endl; // PARALLEL
cout << "Relative position to l3: " << l1.getRelPosTo(l3) << endl; // INTERSECT
return 0;
}
```
注意到我们在函数中使用了 `1e-9` 的精度来比较两个实数是否相等,这是因为浮点数在内存中的存储方式所决定的,相等的两个数可能会略微不同。在实际使用中,我们也可以根据题目要求来调整精度。
阅读全文
相关推荐
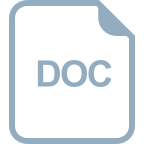
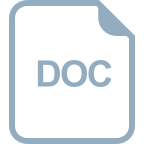
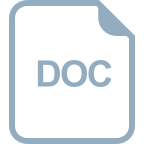
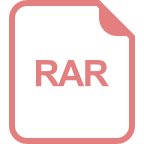
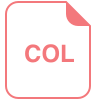

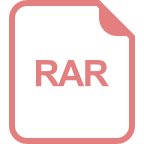
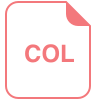
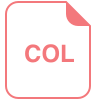
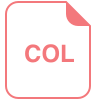
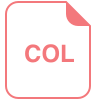
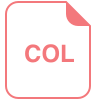
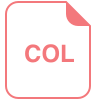
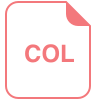
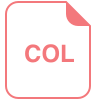

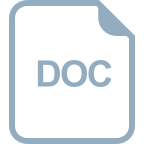