编程实现,在类似于下图的图形化界面下插入记录。1.采用课堂学习中的数据库:xsgl,数据表:student; 2.使用JavaFX搭建所需界面; 3.插入记录前要验证数据的合法性,比如:学号姓名不能空,成绩应在0-100之间; 4.进行基本的测试。
时间: 2024-03-07 21:50:47 浏览: 13
好的,这是一个比较复杂的问题,需要分步骤进行回答。
首先,需要在Java中连接到MySQL数据库,并创建对应的表。可以使用JDBC(Java Database Connectivity)来连接数据库。
其次,需要使用JavaFX来构建图形化界面。JavaFX是Java平台的一部分,用于创建富互联网应用程序(RIA)。
接下来,需要编写Java代码来实现插入记录的功能。在插入记录前,需要进行数据的合法性验证。可以使用正则表达式来验证学号和姓名,使用条件语句来验证成绩是否在0-100之间。
最后,进行基本的测试,包括插入记录的功能测试和数据验证的测试。
以下是大致的代码框架:
```java
import java.sql.*;
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.layout.GridPane;
import javafx.stage.Stage;
public class InsertRecord extends Application {
// JDBC driver name and database URL
static final String JDBC_DRIVER = "com.mysql.jdbc.Driver";
static final String DB_URL = "jdbc:mysql://localhost/xsgl";
// Database credentials
static final String USER = "root";
static final String PASS = "";
@Override
public void start(Stage primaryStage) {
// Create a GridPane for the UI layout
GridPane gridPane = new GridPane();
gridPane.setAlignment(Pos.CENTER);
gridPane.setHgap(10);
gridPane.setVgap(10);
gridPane.setPadding(new Insets(25, 25, 25, 25));
// Add UI controls to the GridPane
Label lblStudentId = new Label("学号:");
gridPane.add(lblStudentId, 0, 0);
TextField txtStudentId = new TextField();
gridPane.add(txtStudentId, 1, 0);
Label lblStudentName = new Label("姓名:");
gridPane.add(lblStudentName, 0, 1);
TextField txtStudentName = new TextField();
gridPane.add(txtStudentName, 1, 1);
Label lblScore = new Label("成绩:");
gridPane.add(lblScore, 0, 2);
TextField txtScore = new TextField();
gridPane.add(txtScore, 1, 2);
Button btnInsert = new Button("插入记录");
gridPane.add(btnInsert, 1, 3);
// Add event handler for the Insert button
btnInsert.setOnAction(event -> {
// Get the input values from the UI controls
String studentId = txtStudentId.getText();
String studentName = txtStudentName.getText();
String scoreStr = txtScore.getText();
// Validate the input values
if (studentId.isEmpty() || studentName.isEmpty() || scoreStr.isEmpty()) {
// Show an error message if any of the input fields is empty
showError("学号、姓名和成绩不能为空!");
} else {
// Validate the score value
int score = Integer.parseInt(scoreStr);
if (score < 0 || score > 100) {
// Show an error message if the score is invalid
showError("成绩必须在0-100之间!");
} else {
// Insert the record into the database
try {
// Register JDBC driver
Class.forName(JDBC_DRIVER);
// Open a connection to the database
Connection conn = DriverManager.getConnection(DB_URL, USER, PASS);
// Create a statement object
Statement stmt = conn.createStatement();
// Construct an SQL statement to insert the record
String sql = "INSERT INTO student (student_id, student_name, score) " +
"VALUES ('" + studentId + "', '" + studentName + "', " + score + ")";
// Execute the SQL statement
stmt.executeUpdate(sql);
// Close the statement and connection objects
stmt.close();
conn.close();
// Show a success message
showSuccess("记录插入成功!");
// Clear the input fields
txtStudentId.setText("");
txtStudentName.setText("");
txtScore.setText("");
} catch (Exception e) {
// Show an error message if an exception occurs
showError("记录插入失败:" + e.getMessage());
}
}
}
});
// Create a Scene and add the GridPane to it
Scene scene = new Scene(gridPane, 400, 300);
// Set the stage title and scene, then show the stage
primaryStage.setTitle("插入记录");
primaryStage.setScene(scene);
primaryStage.show();
}
private void showError(String message) {
// Display an error message in a dialog box
}
private void showSuccess(String message) {
// Display a success message in a dialog box
}
public static void main(String[] args) {
launch(args);
}
}
```
请注意,上述代码仅为示例,需要根据具体情况进行修改和完善。同时,需要注意安全问题,如SQL注入攻击等。
相关推荐
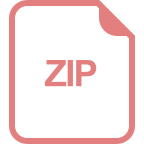
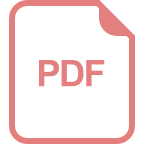
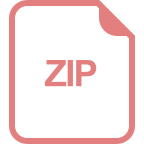








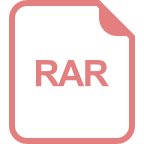