MATlab盲元修复代码
时间: 2023-07-02 22:19:00 浏览: 55
盲元修复是指在没有先验信息的情况下恢复一个信号的缺失部分,MATLAB提供了一些函数来实现这个过程。
以下是一个简单的盲元修复代码示例:
```matlab
% 假设我们有一个长度为N的信号x,其中有M个元素是缺失的
N = 1000;
M = 200;
x = randn(N,1); % 生成一个随机的信号
missing_idx = sort(randperm(N,M)); % 随机选择M个位置作为缺失位置
x(missing_idx) = NaN; % 将缺失位置设为NaN
% 使用MATLAB自带的函数进行盲元修复
x_recovered = inpaintn(x); % 使用inpaintn函数进行修复
% 绘制结果
figure;
plot(1:N,x,'b',1:N,x_recovered,'r');
legend('Original','Recovered');
```
这个代码使用了MATLAB自带的`inpaintn`函数进行盲元修复。`inpaintn`函数可以自动推断信号中缺失部分的值。在这个例子中,我们生成了一个长度为1000的随机信号,并随机选择了200个位置作为缺失位置。然后使用`inpaintn`函数对这个信号进行修复,并绘制了修复前后的对比图。
相关问题
matlab盲元修复代码
盲元修复是指在没有先验信息的情况下恢复一个信号的缺失部分,MATLAB提供了一些函数来实现这个过程。
以下是一个简单的盲元修复代码示例:
```matlab
% 假设我们有一个长度为N的信号x,其中有M个元素是缺失的
N = 1000;
M = 200;
x = randn(N,1); % 生成一个随机的信号
missing_idx = sort(randperm(N,M)); % 随机选择M个位置作为缺失位置
x(missing_idx) = NaN; % 将缺失位置设为NaN
% 使用MATLAB自带的函数进行盲元修复
x_recovered = inpaintn(x); % 使用inpaintn函数进行修复
% 绘制结果
figure;
plot(1:N,x,'b',1:N,x_recovered,'r');
legend('Original','Recovered');
```
这个代码使用了MATLAB自带的`inpaintn`函数进行盲元修复。`inpaintn`函数可以自动推断信号中缺失部分的值。在这个例子中,我们生成了一个长度为1000的随机信号,并随机选择了200个位置作为缺失位置。然后使用`inpaintn`函数对这个信号进行修复,并绘制了修复前后的对比图。
matlab 盲元簇修复代码
盲元簇修复算法是一种基于稀疏表示的图像修复算法,可以用于恢复图像中的缺失区域。以下是一个简单的Matlab代码示例:
```matlab
% 读入含有缺失区域的图像
img = imread('missing_area_image.jpg');
[m, n, ~] = size(img);
% 将图像转为列向量
img_vec = reshape(img, [], n);
% 设置字典大小和稀疏度
dict_size = 100;
sparsity = 5;
% 使用K-SVD算法学习字典
[D, X] = ksvd(img_vec', dict_size, sparsity);
% 选择缺失区域的样本
sample = img_vec(:, 100:200);
% 使用OMP算法求解稀疏表示
X_sample = omp(D, sample, [], sparsity);
% 使用稀疏表示恢复缺失区域
reconstructed_sample = D * X_sample;
img_vec(:, 100:200) = reconstructed_sample;
% 将列向量转为图像
reconstructed_img = reshape(img_vec, m, n, []);
% 显示恢复后的图像
imshow(reconstructed_img);
```
这段代码中使用了K-SVD算法学习字典,并使用OMP算法求解稀疏表示,最后使用稀疏表示恢复缺失区域。需要注意的是,这只是一个简单的示例,实际应用中还需要对算法进行参数调优以获得更好的恢复效果。
相关推荐
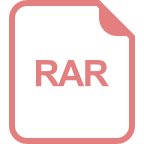
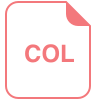
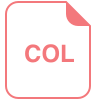
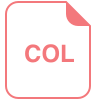
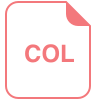
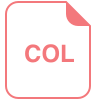







