android+studio俄罗斯方块
时间: 2023-12-11 22:33:45 浏览: 50
Android Studio是一款非常流行的Android应用程序开发工具,可以用于开发各种类型的应用程序,包括游戏。俄罗斯方块是一种非常受欢迎的游戏,可以在Android Studio中使用Java编写。以下是实现俄罗斯方块游戏的一些步骤:
1. 创建一个新的Android Studio项目,并设置项目名称和包名。
2. 在项目中创建一个新的Activity,并将其设置为主Activity。
3. 在布局文件中添加一个SurfaceView,用于绘制游戏界面。
4. 创建一个Game类,用于处理游戏逻辑和绘制游戏界面。
5. 在Game类中实现游戏逻辑,包括方块的移动、旋转和消除等。
6. 在Game类中实现绘制游戏界面的方法,包括绘制背景墙、方块和控制面板等。
7. 在MainActivity中创建一个Game对象,并将其与SurfaceView关联。
8. 在MainActivity中实现游戏控制逻辑,包括处理用户输入和更新游戏状态等。
下面是一个简单的示例代码,用于实现俄罗斯方块游戏:
```java
public class Game {
private int[][] board;
private Block currentBlock;
private int score;
public Game() {
board = new int[10][20];
currentBlock = new Block();
score = 0;
}
public void update() {
if (currentBlock.canMoveDown(board)) {
currentBlock.moveDown();
} else {
currentBlock.addToBoard(board);
int lines = clearLines();
score += lines * 100;
currentBlock = new Block();
}
}
public void moveLeft() {
if (currentBlock.canMoveLeft(board)) {
currentBlock.moveLeft();
}
}
public void moveRight() {
if (currentBlock.canMoveRight(board)) {
currentBlock.moveRight();
}
}
public void rotate() {
if (currentBlock.canRotate(board)) {
currentBlock.rotate();
}
}
public int getScore() {
return score;
}
public int[][] getBoard() {
return board;
}
private int clearLines() {
int lines = 0;
for (int i = 0; i < board[0].length; i++) {
boolean full = true;
for (int j = 0; j < board.length; j++) {
if (board[j][i] == 0) {
full = false;
break;
}
}
if (full) {
lines++;
for (int j = 0; j < board.length; j++) {
for (int k = i; k > 0; k--) {
board[j][k] = board[j][k - 1];
}
board[j][0] = 0;
}
}
}
return lines;
}
}
public class Block {
private int[][] shape;
private int x;
private int y;
public Block() {
Random random = new Random();
int type = random.nextInt(7);
switch (type) {
case 0:
shape = new int[][]{{1, 1}, {1, 1}};
break;
case 1:
shape = new int[][]{{0, 1, 0}, {1, 1, 1}};
break;
case 2:
shape = new int[][]{{0, 1, 1}, {1, 1, 0}};
break;
case 3:
shape = new int[][]{{1, 1, 0}, {0, 1, 1}};
break;
case 4:
shape = new int[][]{{1, 0, 0}, {1, 1, 1}};
break;
case 5:
shape = new int[][]{{0, 0, 1}, {1, 1, 1}};
break;
case 6:
shape = new int[][]{{1, 1, 1, 1}};
break;
}
x = 4;
y = 0;
}
public boolean canMoveDown(int[][] board) {
for (int i = 0; i < shape.length; i++) {
for (int j = 0; j < shape[i].length; j++) {
if (shape[i][j] != 0) {
int row = y + i + 1;
int col = x + j;
if (row >= board[0].length || board[col][row] != 0) {
return false;
}
}
}
}
return true;
}
public void moveDown() {
y++;
}
public boolean canMoveLeft(int[][] board) {
for (int i = 0; i < shape.length; i++) {
for (int j = 0; j < shape[i].length; j++) {
if (shape[i][j] != 0) {
int row = y + i;
int col = x + j - 1;
if (col < 0 || board[col][row] != 0) {
return false;
}
}
}
}
return true;
}
public void moveLeft() {
x--;
}
public boolean canMoveRight(int[][] board) {
for (int i = 0; i < shape.length; i++) {
for (int j = 0; j < shape[i].length; j++) {
if (shape[i][j] != 0) {
int row = y + i;
int col = x + j + 1;
if (col >= board.length || board[col][row] != 0) {
return false;
}
}
}
}
return true;
}
public void moveRight() {
x++;
}
public boolean canRotate(int[][] board) {
int[][] newShape = new int[shape[0].length][shape.length];
for (int i = 0; i < shape.length; i++) {
for (int j = 0; j < shape[i].length; j++) {
newShape[j][shape.length - 1 - i] = shape[i][j];
}
}
for (int i = 0; i < newShape.length; i++) {
for (int j = 0; j < newShape[i].length; j++) {
if (newShape[i][j] != 0) {
int row = y + i;
int col = x + j;
if (row < 0 || row >= board[0].length || col < 0 || col >= board.length || board[col][row] != 0) {
return false;
}
}
}
}
shape = newShape;
return true;
}
public void rotate() {
int[][] newShape = new int[shape[0].length][shape.length];
for (int i = 0; i < shape.length; i++) {
for (int j = 0; j < shape[i].length; j++) {
newShape[j][shape.length - 1 - i] = shape[i][j];
}
}
shape = newShape;
}
public void addToBoard(int[][] board) {
for (int i = 0; i < shape.length; i++) {
for (int j = 0; j < shape[i].length; j++) {
if (shape[i][j] != 0) {
board[x + j][y + i] = shape[i][j];
}
}
}
}
public int[][] getShape() {
return shape;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
}
public class GameView extends SurfaceView implements SurfaceHolder.Callback {
private GameThread thread;
private Game game;
public GameView(Context context, AttributeSet attrs) {
super(context, attrs);
getHolder().addCallback(this);
thread = new GameThread(getHolder(), this);
setFocusable(true);
}
@Override
public void surfaceCreated(SurfaceHolder holder) {
game = new Game();
thread.setRunning(true);
thread.start();
}
@Override
public void surfaceChanged(SurfaceHolder holder, int format, int width, int height) {
}
@Override
public void surfaceDestroyed(SurfaceHolder holder) {
boolean retry = true;
while (retry) {
try {
thread.setRunning(false);
thread.join();
retry = false;
} catch (InterruptedException e) {
}
}
}
@Override
public boolean onTouchEvent(MotionEvent event) {
if (event.getAction() == MotionEvent.ACTION_DOWN) {
float x = event.getX();
float y = event.getY();
if (x < getWidth() / 2) {
game.moveLeft();
} else {
game.moveRight();
}
}
return true;
}
public void update() {
game.update();
}
@Override
public void draw(Canvas canvas) {
super.draw(canvas);
if (canvas != null) {
int[][] board = game.getBoard();
int blockSize = getWidth() / 10;
Paint paint = new Paint();
paint.setStyle(Paint.Style.FILL);
for (int i = 0; i < board.length; i++) {
for (int j = 0; j < board[i].length; j++) {
if (board[i][j] != 0) {
paint.setColor(getColor(board[i][j]));
canvas.drawRect(i * blockSize, j * blockSize, (i + 1) * blockSize, (j + 1) * blockSize, paint);
}
}
}
Block currentBlock = game.getCurrentBlock();
int[][] shape = currentBlock.getShape();
int x = currentBlock.getX();
int y = currentBlock.getY();
for (int i = 0; i < shape.length; i++) {
for (int j = 0; j < shape[i].length; j++) {
if (shape[i][j] != 0) {
paint.setColor(getColor(shape[i][j]));
canvas.drawRect((x + j) * blockSize, (y + i) * blockSize, (x + j + 1) * blockSize, (y + i + 1) * blockSize, paint);
}
}
}
}
}
private int getColor(int value) {
switch (value) {
case 1:
return Color.RED;
case 2:
return Color.GREEN;
case 3:
return Color.BLUE;
case 4:
return Color.YELLOW;
case 5:
return Color.CYAN;
case 6:
return Color.MAGENTA;
case 7:
return Color.GRAY;
default:
return Color.WHITE;
}
}
}
public class GameThread extends Thread {
private SurfaceHolder surfaceHolder;
private GameView gameView;
private boolean running;
public GameThread(SurfaceHolder surfaceHolder, GameView gameView) {
this.surfaceHolder = surfaceHolder;
this.gameView = gameView;
}
public void setRunning(boolean running) {
this.running = running;
}
@Override
public void run() {
while (running) {
Canvas canvas = null;
try {
canvas = surfaceHolder.lockCanvas();
synchronized (surfaceHolder) {
gameView.update();
gameView.draw(canvas);
}
} finally {
if (canvas != null) {
surfaceHolder.unlockCanvasAndPost(canvas);
}
}
}
}
}
MainActivity中的代码:
public class MainActivity extends AppCompatActivity {
private GameView gameView;
private TextView scoreView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
gameView = findViewById(R.id.game_view);
scoreView = findViewById(R.id.score_view);
gameView.setScoreView(scoreView);
}
}
activity_main.xml中的代码:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<com.example.tetris.GameView
android:id="@+id/game_view"
android:layout_width="match_parent"
android:layout_height="match_parent" />
<TextView
android:id="@+id/score_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Score: 0"
android:textSize="24sp"
android:textStyle="bold"
android:layout_alignParentTop="true"
android:layout_alignParentEnd="true"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp" />
</RelativeLayout>
--相关问题--:
相关推荐
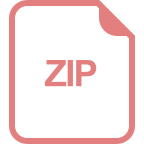
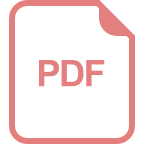














