java批量修改数据库数据
时间: 2023-10-04 21:05:55 浏览: 97
首先,你需要编写一个 Java 程序来连接数据库。可以使用 JDBC API 来实现这一点。接下来,你需要编写 SQL 查询语句来选择要修改的数据。然后,你可以使用 Java 代码来批量更新数据库中的数据。
下面是一个简单的示例程序,演示了如何使用 Java 批量更新数据库中的数据:
```java
import java.sql.*;
public class BatchUpdateExample {
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
try {
// 连接数据库
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection("jdbc:mysql://localhost/mydatabase", "username", "password");
// 创建 Statement 对象
stmt = conn.createStatement();
// 执行批量更新操作
stmt.addBatch("UPDATE mytable SET column1 = 'newvalue1' WHERE id = 1");
stmt.addBatch("UPDATE mytable SET column2 = 'newvalue2' WHERE id = 2");
stmt.addBatch("UPDATE mytable SET column3 = 'newvalue3' WHERE id = 3");
stmt.executeBatch();
System.out.println("批量更新成功!");
} catch (ClassNotFoundException | SQLException e) {
e.printStackTrace();
} finally {
// 关闭数据库连接
try {
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
在上面的示例中,我们使用了 MySQL 数据库,并执行了三个批量更新操作。你需要将其中的数据库连接信息和 SQL 查询语句替换为你自己的。
阅读全文
相关推荐
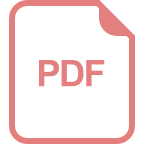
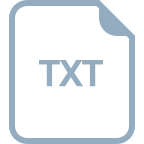
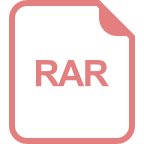
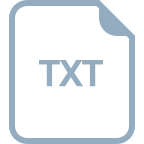
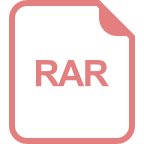
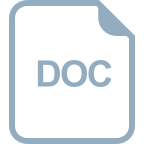
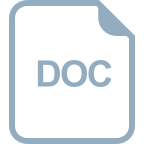






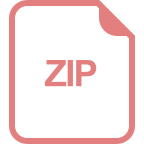
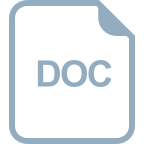