统计一个文本文件ex1.txt中字母、数字各有多少个
时间: 2024-04-12 15:28:46 浏览: 219
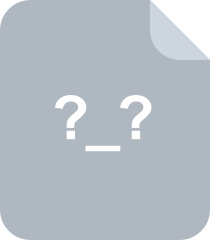
统计文件中字母的个数
你可以使用以下代码来统计一个文本文件中字母和数字的数量:
```python
def count_chars_digits(filename):
with open(filename, 'r') as file:
text = file.read()
chars = sum(c.isalpha() for c in text)
digits = sum(c.isdigit() for c in text)
return chars, digits
filename = 'ex1.txt'
char_count, digit_count = count_chars_digits(filename)
print("字母数量:", char_count)
print("数字数量:", digit_count)
```
请确保将文件名替换为你的实际文件名。此代码将打开文件并读取其中的文本。然后,它将使用isalpha()和isdigit()方法来判断每个字符是字母还是数字,并对它们进行计数。最后,它将打印出字母和数字的数量。
阅读全文
相关推荐
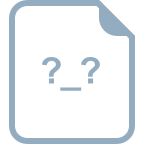

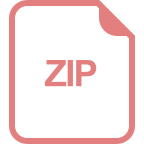
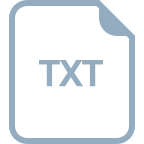




