初始化顺序表list,将图书信息输入顺序表,然后遍历顺序表;读取第4个元素的值;查找价格是38的图书信息;在第4个元素前插入元素,然后遍历顺序表;删除第7个元素,遍历顺序表
时间: 2024-09-13 16:15:50 浏览: 45
初始化顺序表(如C++的vector或Python的列表)通常涉及创建一个新的容器,并分配适当的内存来存储数据。例如,在C++中,你可以这样做:
```cpp
#include <vector>
std::vector<std::pair<int, string>> bookList; // 书籍信息包含ID和名称
```
为了向顺序表添加图书信息,你需要遍历它(如果为空),或者找到合适的位置插入新元素:
```cpp
// 添加图书
std::pair<int, string> newBook(42, "《计算机基础》");
size_t index = bookList.size(); // 如果已满则默认追加到末尾
bookList.insert(bookList.begin() + index, newBook);
```
遍历顺序表可以这样操作:
```cpp
for (const auto& book : bookList) {
std::cout << "ID: " << book.first << ", Title: " << book.second << "\n";
}
```
读取第四个元素的值:
```cpp
auto fourthBook = bookList[3];
std::cout << "Fourth book ID: " << fourthBook.first << ", Title: " << fourthBook.second << "\n";
```
查找价格为38的图书:
```cpp
for (auto it = bookList.begin(); it != bookList.end(); ++it) {
if (it->first == 38) {
std::cout << "Found book with price 38: " << it->second << "\n";
break;
}
}
```
在第四个元素前插入元素:
```cpp
std::pair<int, string> insertElement(35, "《算法导论》");
bookList.insert(bookList.begin() + 3, insertElement);
```
最后,删除第七个元素(注意索引是从0开始计数的,所以第七个元素的索引是6):
```cpp
if (bookList.size() > 6) {
bookList.erase(bookList.begin() + 6);
}
```
再次遍历顺序表以确认更改:
```cpp
for (const auto& book : bookList) {
// ...
}
```
阅读全文
相关推荐
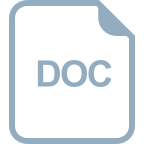
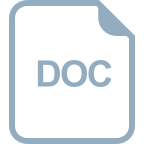
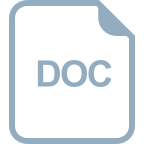



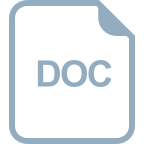
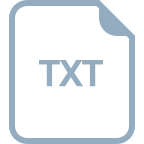
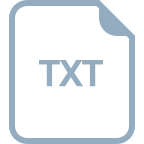
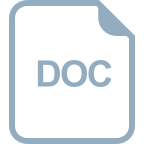
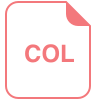







