c/c++ 经纬度转国家2000 坐标算法 demo
时间: 2024-09-24 21:12:19 浏览: 32
经纬度转换成国家名称通常涉及地理编码服务,这涉及到复杂的地理位置数据处理和数据库查询。C/C++本身并不直接提供这种功能,因为它们主要用于系统编程和底层操作,而不是地图API。你需要使用专门的地理定位库,如GDAL、Geospatial Data Abstraction Library 或者第三方地图服务提供的API,比如Google Maps API、OpenStreetMap Nominatim等。
以下是一个简单的伪代码示例,展示了如何使用C++配合外部库来进行这个过程:
```cpp
#include <geos/geos.h>
#include <string>
// 假设你有一个包含经度和纬度的结构体或变量
struct Coordinate {
double latitude;
double longitude;
};
std::string coordinate_to_country(Coordinate coord) {
// 创建GeoJSON Point对象
GEOSGeometry* point = GEOSGeom_createPoint(GEOSDoubleToRadian(coord.longitude), GEOSDoubleToRadian(coord.latitude));
// 使用Nominatim或其他地理编码库进行查询
std::string country;
if (geocode_with_nominatim(point, &country)) {
// 如果成功获取到国家名,则返回
return country;
} else {
// 处理错误或无法找到对应国家的情况
return "Country not found";
}
// 清理GEOS对象
delete point;
}
bool geocode_with_nominatim(GEOSGeometry* point, std::string* country) {
// 这里只是一个简化的接口,实际需要连接到Nominatim API或者其他地理编码服务
// 实际代码会发送HTTP请求并解析响应
// 示例:(假设有个函数geoname_search调用了Nominatim API)
*country = geoname_search(point->getEnvelope(), "json");
// 检查API是否成功返回了结果
if (!country->empty()) {
return true;
}
return false;
}
// 示例用法
int main() {
Coordinate coord = {48.8566, 2.3522}; // 巴黎坐标
std::string country_name = coordinate_to_country(coord);
std::cout << "Country for the coordinates: " << country_name << std::endl;
return 0;
}
```
注意:此代码仅作为概念演示,并未包含完整的地理编码库集成,你需要实际查找一个合适的C++地理编码库,并按照其文档调整代码。此外,使用外部API可能会涉及网络请求和版权问题,务必遵守相关条款。
相关推荐
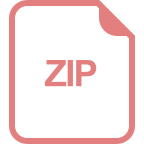
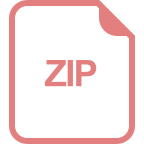
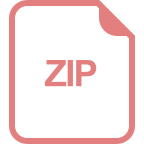














