请介绍如何利用Python实现一个基于序列标注的命名实体识别(NER)系统,并附上一段具体的实现代码。
时间: 2024-12-04 07:16:23 浏览: 22
要实现一个基于序列标注的命名实体识别(NER)系统,你可以采用诸如 Conditional Random Fields (CRF) 或 LSTM-CRF 这样的模型。《NLP毕设项目:实体与关系联合抽取的Python实现》是直接针对这一主题的实用资源,它不仅提供了详细的Python源码实现,还包括了文档说明,让你能够快速掌握从零开始构建NER系统的过程。下面是一个简单的NER系统实现示例,使用了BiLSTM-CRF模型:
参考资源链接:[NLP毕设项目:实体与关系联合抽取的Python实现](https://wenku.csdn.net/doc/14mup9xdte?spm=1055.2569.3001.10343)
import torch
from torchcrf import CRF
from allennlp.modules.conditional_random_field import ConditionalRandomField, allowed_transitions
# 假设你已经有了预处理后的数据,以及相应的词汇表
# 数据预处理可能包括分词、构建词汇表、将文本数据转换为整数索引等步骤
# 定义模型结构
class BiLSTM_CRF(torch.nn.Module):
def __init__(self, vocab_size, tag_to_ix, embedding_dim, hidden_dim):
super(BiLSTM_CRF, self).__init__()
self.embedding_dim = embedding_dim
self.hidden_dim = hidden_dim
self.vocab_size = vocab_size
self.tag_to_ix = tag_to_ix
self.tagset_size = len(tag_to_ix)
self.word_embeds = torch.nn.Embedding(vocab_size, embedding_dim)
self.lstm = torch.nn.LSTM(embedding_dim, hidden_dim // 2,
num_layers=1, bidirectional=True)
# Maps the output of the LSTM into tag space.
self.hidden2tag = torch.nn.Linear(hidden_dim, self.tagset_size)
# CRF layer
self.crf = CRF(self.tagset_size)
def forward(self, sentence):
# Get the emission scores from the BiLSTM
embeds = self.word_embeds(sentence).view(len(sentence), 1, -1)
lstm_out, _ = self.lstm(embeds)
lstm_feats = self.hidden2tag(lstm_out)
# Find the best path, given the features.
score, tag_seq = self.crf(lstm_feats)
return score, tag_seq
# 初始化模型
model = BiLSTM_CRF(vocab_size=10000, tag_to_ix=tag_to_ix,
embedding_dim=128, hidden_dim=256)
# 定义优化器
optimizer = torch.optim.SGD(model.parameters(), lr=0.01, weight_decay=1e-4)
# 训练模型(省略了训练代码,包含前向传播、反向传播、优化等步骤)
# 假设你已经加载了训练好的模型参数
# 使用模型进行预测
def predict(sentence):
model.eval()
with torch.no_grad():
inputs = torch.tensor(sentence, dtype=torch.long)
score, tag_seq = model(inputs)
return score, tag_seq
# 示例句子
example_sentence = [list_of_word_indices] # 需要将词语转换为索引
# 进行预测
score, tag_seq = predict(example_sentence)
print(tag_seq)
# 注意:在实际使用中,你需要加载预处理后的数据、模型参数,以及编写完整训练循环。此外,还应进行模型评估,查看其在验证集或测试集上的表现。
在学习了如何使用《NLP毕设项目:实体与关系联合抽取的Python实现》这一资源后,如果你想进一步深入研究NER,建议继续查看《Information Extraction with BiLSTM-CRFs》,这本书提供了关于如何使用BiLSTM-CRF进行信息抽取的更多细节和高级技术。
参考资源链接:[NLP毕设项目:实体与关系联合抽取的Python实现](https://wenku.csdn.net/doc/14mup9xdte?spm=1055.2569.3001.10343)
阅读全文
相关推荐
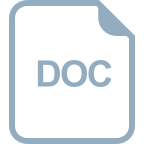
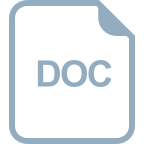
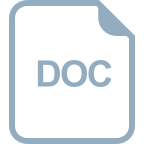

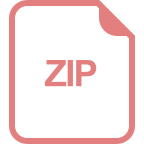
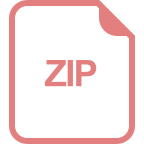
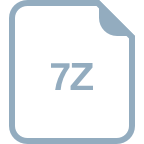
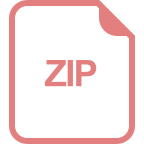
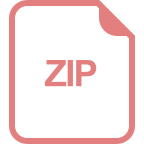
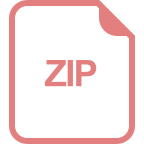
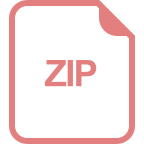
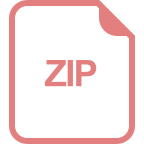
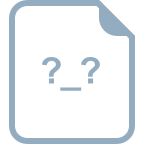
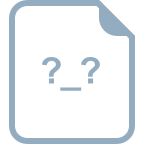
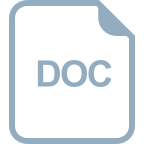



