编写一个程序统计十个同学的最高分, 最低分以及平均分
时间: 2024-11-27 18:13:38 浏览: 5
为了帮助您编写一个简单的Java程序来统计十位同学的成绩,我们可以创建一个名为`Student`的类,其中包含每个人的分数,然后创建一个`ScoreAnalyzer`类来处理这些数据。这里有一个基本的例子:
```java
// Student 类表示一位学生及其分数
class Student {
private String name;
private int score;
public Student(String name, int score) {
this.name = name;
this.score = score;
}
// 获取名字和分数的方法
public String getName() {
return name;
}
public int getScore() {
return score;
}
}
// ScoreAnalyzer 类用于计算最高分、最低分和平均分
public class ScoreAnalyzer {
private List<Student> students;
public ScoreAnalyzer(List<Student> students) {
this.students = students;
}
// 计算最高分
public int getMaxScore() {
if (students.isEmpty()) {
throw new IllegalArgumentException("No students found.");
}
return Collections.max(students.stream().mapToInt(Student::getScore).boxed(), Integer::compareTo);
}
// 计算最低分
public int getMinScore() {
if (students.isEmpty()) {
throw new IllegalArgumentException("No students found.");
}
return Collections.min(students.stream().mapToInt(Student::getScore).boxed(), Integer::compareTo);
}
// 计算平均分
public double getAverageScore() {
if (students.isEmpty()) {
throw new IllegalArgumentException("No students found.");
}
double sum = students.stream().mapToDouble(Student::getScore).sum();
return sum / students.size();
}
}
// 主函数
public class Main {
public static void main(String[] args) {
// 创建十个学生实例并添加到列表中
List<Student> students = new ArrayList<>();
students.add(new Student("Alice", 85));
students.add(new Student("Bob", 90));
students.add(new Student("Charlie", 80));
// ...(继续添加其他7个学生的分数)
// 使用ScoreAnalyzer分析数据
ScoreAnalyzer analyzer = new ScoreAnalyzer(students);
try {
int maxScore = analyzer.getMaxScore();
int minScore = analyzer.getMinScore();
double averageScore = analyzer.getAverageScore();
System.out.println("Max score: " + maxScore);
System.out.println("Min score: " + minScore);
System.out.println("Average score: " + averageScore);
} catch (IllegalArgumentException e) {
System.out.println(e.getMessage());
}
}
}
```
这个例子中,我们首先定义了`Student`类,然后在`ScoreAnalyzer`类中实现了统计功能。在`main`函数里,我们创建了一个包含10个学生的列表,并调用`ScoreAnalyzer`进行计算。
阅读全文
相关推荐
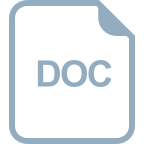
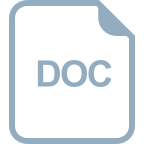















