c#dictionary读写操作
时间: 2024-08-14 14:10:14 浏览: 82
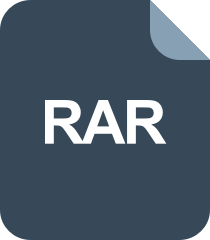
C# 读写INI 实例源码(系统操作)
在C#中,Dictionary是一种哈希表数据结构,用于存储键值对。它的读写操作非常直观和高效。下面是一些基本的操作:
1. **读取(Get)**:
- 如果字典中存在指定的键,`Dictionary<TKey, TValue>.this[TKey key]`表达式可以获取对应的值。例如:
```csharp
Dictionary<string, int> dict = new Dictionary<string, int>();
int value = dict["key"]; // 如果"key"存在,返回其对应的整数值,否则抛出异常
```
2. **写入(Add)**:
- 使用`Add(KeyValuePair<TKey, TValue> item)`方法添加新的键值对。例如:
```csharp
dict.Add("newKey", 42);
```
或者单独添加键和值:
```csharp
dict["anotherKey"] = 99;
```
3. **更新(Update)**:
- 对于已存在的键,可以直接通过索引修改值,如:
```csharp
dict["existingKey"] = newValue;
```
4. **删除(Remove)**:
- 可以使用`Remove(TKey key)`方法移除指定键的项,如果不存在则不会影响。
```csharp
dict.Remove("toBeRemoved");
```
5. **遍历(Iteration)**:
- 可以通过`foreach`循环遍历所有键值对:
```csharp
foreach (KeyValuePair<string, int> pair in dict)
Console.WriteLine($"Key: {pair.Key}, Value: {pair.Value}");
```
6. **序列化与反序列化(Serialization and Deserialization)**:
- 使用`JsonConvert.SerializeObject()`将Dictionary转换为JSON字符串,或使用`JsonConvert.DeserializeObject()`从JSON字符串恢复。
阅读全文
相关推荐
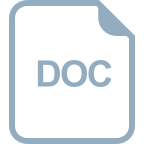
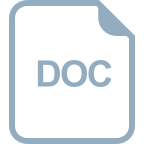
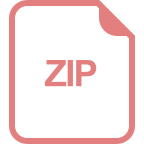
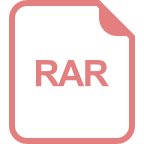
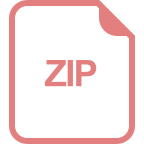
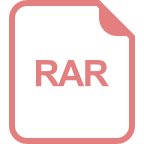
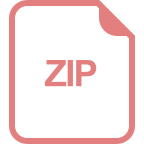
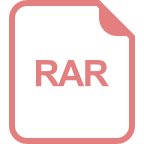
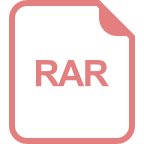
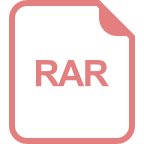
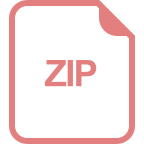
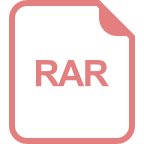
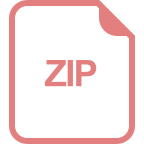
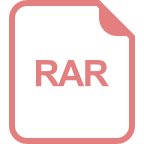
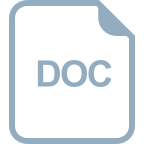
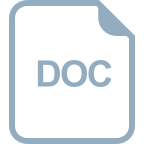
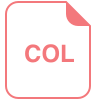
