es.indices.get_alias
时间: 2023-10-23 21:10:52 浏览: 173
你好!关于获取索引别名的问题,您可以使用 Elasticsearch 的 `indices.get_alias` API 来获取。这个 API 接受一个别名(alias)作为参数,并返回与该别名相关联的索引信息。
以下是一个示例请求:
```python
import elasticsearch
from elasticsearch import Elasticsearch
# 创建 Elasticsearch 客户端
client = Elasticsearch()
# 获取别名为 "my_alias" 的索引信息
response = client.indices.get_alias(name="my_alias")
# 输出结果
print(response)
```
请注意,您需要先安装 `elasticsearch` Python 模块,以及在代码中适当配置 Elasticsearch 客户端的连接信息(如主机地址、端口等)。
希望这可以回答您的问题!如果您还有其他疑问,请随时提出。
相关问题
curl -XGET "http://localhost:9200/your_index/_mapping?pretty" { "error" : { "root_cause" : [ { "type" : "index_not_found_exception", "reason" : "no such index [your_index]", "resource.type" : "index_or_alias", "resource.id" : "your_index", "index_uuid" : "_na_", "index" : "your_index" } ], "type" : "index_not_found_exception", "reason" : "no such index [your_index]", "resource.type" : "index_or_alias", "resource.id" : "your_index", "index_uuid" : "_na_", "index" : "your_index" }, "status" : 404 }
这个错误提示表明 Elasticsearch 中不存在名为 `your_index` 的索引。这可能是由于以下原因之一导致的:
1. 索引未创建。请确认你是否已经正确创建了 `your_index` 索引。你可以使用以下命令来检查索引是否存在:`curl -XGET "http://localhost:9200/_cat/indices?v"`
2. 索引名称拼写错误。请检查你的命令中使用的索引名称是否正确拼写为 `your_index`。
你可以通过以下步骤来解决问题:
1. 确认你已经正确创建了 `your_index` 索引。如果没有,请使用以下命令在 Elasticsearch 中创建索引:`curl -XPUT "http://localhost:9200/your_index"`
2. 如果你发现索引名称拼写错误,可以在命令中进行更正,并重新执行命令。
如果你仍然无法解决问题,请提供更多的详细信息和错误日志,以便我更好地帮助你解决问题。
elasticsearch通用工具类
以下是一个基本的 Elasticsearch 工具类,可以用于与 Elasticsearch 交互:
```java
import org.apache.http.HttpHost;
import org.elasticsearch.action.bulk.BulkRequest;
import org.elasticsearch.action.bulk.BulkResponse;
import org.elasticsearch.action.delete.DeleteRequest;
import org.elasticsearch.action.delete.DeleteResponse;
import org.elasticsearch.action.get.GetRequest;
import org.elasticsearch.action.get.GetResponse;
import org.elasticsearch.action.index.IndexRequest;
import org.elasticsearch.action.index.IndexResponse;
import org.elasticsearch.action.search.SearchRequest;
import org.elasticsearch.action.search.SearchResponse;
import org.elasticsearch.client.RestClient;
import org.elasticsearch.client.RestHighLevelClient;
import org.elasticsearch.common.xcontent.XContentType;
import org.elasticsearch.index.query.QueryBuilder;
import org.elasticsearch.index.query.QueryBuilders;
import org.elasticsearch.search.SearchHit;
import org.elasticsearch.search.builder.SearchSourceBuilder;
import java.io.IOException;
import java.util.List;
import java.util.Map;
public class ElasticsearchUtil {
private RestHighLevelClient client;
public ElasticsearchUtil(String host, int port) {
client = new RestHighLevelClient(RestClient.builder(new HttpHost(host, port, "http")));
}
public void close() throws IOException {
client.close();
}
public boolean indexExists(String index) throws IOException {
return client.indices().exists(new GetIndexRequest(index), RequestOptions.DEFAULT);
}
public boolean createIndex(String index) throws IOException {
CreateIndexRequest request = new CreateIndexRequest(index);
request.settings(Settings.builder()
.put("index.number_of_shards", 3)
.put("index.number_of_replicas", 2)
);
request.mapping("properties", "{\n" +
" \"name\": {\n" +
" \"type\": \"text\"\n" +
" },\n" +
" \"age\": {\n" +
" \"type\": \"integer\"\n" +
" }\n" +
"}", XContentType.JSON);
request.alias(new Alias("my_alias"));
CreateIndexResponse response = client.indices().create(request, RequestOptions.DEFAULT);
return response.isAcknowledged();
}
public boolean deleteIndex(String index) throws IOException {
DeleteIndexRequest request = new DeleteIndexRequest(index);
AcknowledgedResponse response = client.indices().delete(request, RequestOptions.DEFAULT);
return response.isAcknowledged();
}
public boolean documentExists(String index, String id) throws IOException {
GetRequest getRequest = new GetRequest(index, id);
getRequest.fetchSourceContext(new FetchSourceContext(false));
getRequest.storedFields("_none_");
return client.exists(getRequest, RequestOptions.DEFAULT);
}
public boolean createDocument(String index, String id, Map<String, Object> source) throws IOException {
IndexRequest request = new IndexRequest(index);
if (id != null) {
request.id(id);
}
request.source(source);
IndexResponse response = client.index(request, RequestOptions.DEFAULT);
return response.getResult() == DocWriteResponse.Result.CREATED;
}
public boolean updateDocument(String index, String id, Map<String, Object> source) throws IOException {
UpdateRequest request = new UpdateRequest(index, id);
request.doc(source);
UpdateResponse response = client.update(request, RequestOptions.DEFAULT);
return response.getResult() == DocWriteResponse.Result.UPDATED;
}
public boolean deleteDocument(String index, String id) throws IOException {
DeleteRequest request = new DeleteRequest(index, id);
DeleteResponse response = client.delete(request, RequestOptions.DEFAULT);
return response.getResult() == DocWriteResponse.Result.DELETED;
}
public Map<String, Object> getDocument(String index, String id) throws IOException {
GetRequest getRequest = new GetRequest(index, id);
GetResponse response = client.get(getRequest, RequestOptions.DEFAULT);
if (response.isExists()) {
return response.getSource();
} else {
return null;
}
}
public List<Map<String, Object>> searchDocument(String index, String field, String value) throws IOException {
QueryBuilder queryBuilder = QueryBuilders.matchQuery(field, value);
SearchSourceBuilder sourceBuilder = new SearchSourceBuilder();
sourceBuilder.query(queryBuilder);
SearchRequest searchRequest = new SearchRequest(index);
searchRequest.source(sourceBuilder);
SearchResponse searchResponse = client.search(searchRequest, RequestOptions.DEFAULT);
List<Map<String, Object>> result = new ArrayList<>();
for (SearchHit hit : searchResponse.getHits().getHits()) {
result.add(hit.getSourceAsMap());
}
return result;
}
public boolean bulkCreateDocuments(String index, List<Map<String, Object>> sources) throws IOException {
BulkRequest request = new BulkRequest();
for (Map<String, Object> source : sources) {
IndexRequest indexRequest = new IndexRequest(index);
indexRequest.source(source);
request.add(indexRequest);
}
BulkResponse response = client.bulk(request, RequestOptions.DEFAULT);
return !response.hasFailures();
}
public boolean bulkUpdateDocuments(String index, List<Map<String, Object>> sources) throws IOException {
BulkRequest request = new BulkRequest();
for (Map<String, Object> source : sources) {
String id = (String) source.remove("id");
UpdateRequest updateRequest = new UpdateRequest(index, id);
updateRequest.doc(source);
request.add(updateRequest);
}
BulkResponse response = client.bulk(request, RequestOptions.DEFAULT);
return !response.hasFailures();
}
public boolean bulkDeleteDocuments(String index, List<String> ids) throws IOException {
BulkRequest request = new BulkRequest();
for (String id : ids) {
DeleteRequest deleteRequest = new DeleteRequest(index, id);
request.add(deleteRequest);
}
BulkResponse response = client.bulk(request, RequestOptions.DEFAULT);
return !response.hasFailures();
}
}
```
这个工具类使用 Elasticsearch 的 Java High Level REST Client,支持创建、删除索引,增删改查文档,以及批量操作。
阅读全文
相关推荐
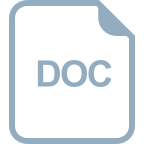
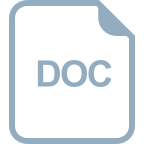
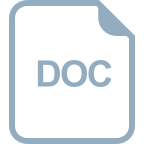
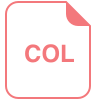
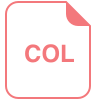
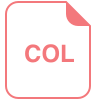
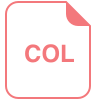
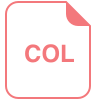
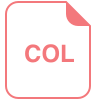
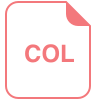
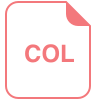
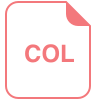
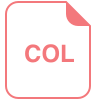
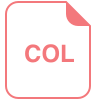


