基于Three.js 绘制3维路线图,并带有坐标系, 请给出完整代码
时间: 2024-03-27 18:37:09 浏览: 16
以下是使用 Three.js 绘制3D路线图并带有坐标系的完整代码示例:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Three.js 3D路线图示例</title>
<style>
body {
margin: 0;
padding: 0;
}
canvas {
width: 100%;
height: 100%;
}
</style>
</head>
<body>
<script src="https://cdn.jsdelivr.net/npm/three@0.131.0/build/three.min.js"></script>
<script>
// 初始化场景、相机、渲染器
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
// 添加坐标系
const axesHelper = new THREE.AxesHelper(5);
scene.add(axesHelper);
// 创建路径点数组
const points = [];
points.push(new THREE.Vector3(-5, 0, 0));
points.push(new THREE.Vector3(-3, 3, 0));
points.push(new THREE.Vector3(0, 5, 0));
points.push(new THREE.Vector3(3, 3, 0));
points.push(new THREE.Vector3(5, 0, 0));
points.push(new THREE.Vector3(3, -3, 0));
points.push(new THREE.Vector3(0, -5, 0));
points.push(new THREE.Vector3(-3, -3, 0));
points.push(new THREE.Vector3(-5, 0, 0));
// 创建路径线条
const geometry = new THREE.BufferGeometry().setFromPoints(points);
const material = new THREE.LineBasicMaterial({ color: 0x0000ff });
const line = new THREE.Line(geometry, material);
scene.add(line);
// 将相机位置设置为路径中心点,使路径居中显示
const center = new THREE.Vector3();
geometry.computeBoundingBox();
geometry.boundingBox.getCenter(center);
camera.position.set(center.x, center.y, 20);
// 添加光源
const light = new THREE.DirectionalLight(0xffffff, 1);
light.position.set(1, 1, 1);
scene.add(light);
// 渲染循环
function animate() {
requestAnimationFrame(animate);
renderer.render(scene, camera);
}
animate();
</script>
</body>
</html>
```
在这个示例中,我们首先初始化了场景、相机和渲染器,然后添加了一个坐标系。接着,我们创建了一个包含多个点的路径数组,并使用 `BufferGeometry` 和 `LineBasicMaterial` 创建了一条路径线条。我们还将相机的位置设置为路径中心点,使路径居中显示,并添加了一个光源。最后,我们开启了渲染循环,实现了动态渲染效果。
相关推荐
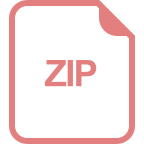
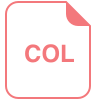
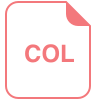
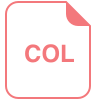
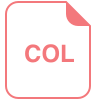
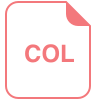









