C++代码:给定一个升序的单链表,只保留独立的元素(int类型
时间: 2024-05-09 20:16:52 浏览: 8
)并且按照升序排序,删除重复的元素。
我理解您需要一个 C 语言代码,该代码可以处理一个给定的升序单链表,只保留独立的元素并且进行升序排序,同时删除重复的元素。以下是该问题的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
struct ListNode {
int data;
struct ListNode* next;
};
void add_node(struct ListNode** head_ref, int new_data) {
struct ListNode* new_node = (struct ListNode*)malloc(sizeof(struct ListNode));
new_node->data = new_data;
new_node->next = (*head_ref);
(*head_ref) = new_node;
}
void remove_duplicates(struct ListNode* head) {
struct ListNode* current = head;
while(current != NULL) {
struct ListNode* runner = current;
while(runner->next != NULL) {
if(current->data == runner->next->data) {
struct ListNode* temp = runner->next;
runner->next = runner->next->next;
free(temp);
} else {
runner = runner->next;
}
}
current = current->next;
}
}
void sort_list(struct ListNode* head) {
int temp;
struct ListNode* temp_node = head;
while(temp_node != NULL) {
struct ListNode* current = temp_node->next;
while(current != NULL) {
if(temp_node->data > current->data) {
temp = temp_node->data;
temp_node->data = current->data;
current->data = temp;
}
current = current->next;
}
temp_node = temp_node->next;
}
}
void print_list(struct ListNode* head) {
struct ListNode* temp_node = head;
while(temp_node != NULL) {
printf("%d ", temp_node->data);
temp_node = temp_node->next;
}
}
int main() {
struct ListNode* head = NULL;
add_node(&head, 8);
add_node(&head, 7);
add_node(&head, 6);
add_node(&head, 6);
add_node(&head, 5);
add_node(&head, 4);
add_node(&head, 3);
add_node(&head, 3);
add_node(&head, 2);
add_node(&head, 1);
sort_list(head);
remove_duplicates(head);
// 输出结果
print_list(head);
return 0;
}
```
该代码首先定义了单向链表结构体(ListNode)。add_node 函数用于添加新的节点到链表中,remove_duplicates 函数用于删除链表中的重复节点,sort_list 函数用于按照升序对链表进行排序,print_list 函数用于将链表中的所有元素打印出来。在 main 函数中,我们先创建一个具有重复元素的升序递归单向链表,然后对其进行排序和删除重复节点,最后打印出链表。
该代码主要思路是通过两个循环,外层循环遍历链表中的每一个元素,把当前元素保存到 current 指针中,然后内层循环从 current 开始遍历所有元素,如果有重复元素,就使用 free() 函数将这些重复元素删除,否则直接跳过。之后再使用另一个循环,将链表进行升序排序。最后通过 print_list 函数将链表中的所有元素打印出来。
希望这段代码可以帮到您,如果还有其他问题,请随时问我。
相关推荐
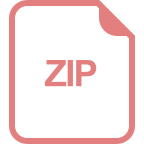
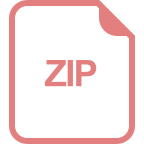














