C#代码IBATIS.net批量更新数据 动态SQL
时间: 2023-09-18 18:06:50 浏览: 66
以下是使用 C# 代码实现 IBatis.NET 动态批量更新数据的示例:
1. 定义 SQL 语句
在 SQL Mapper 文件中定义更新语句,参考上面的示例,并加入动态 SQL。
```xml
<update id="updateBatch">
update user
<dynamic prepend="set">
<isNotEmpty prepend="," property="name">
name = #name#
</isNotEmpty>
<isNotEmpty prepend="," property="age">
age = #age#
</isNotEmpty>
<isNotEmpty prepend="," property="address">
address = #address#
</isNotEmpty>
</dynamic>
where id in
<foreach open="(" close=")" separator="," collection="list" item="item">
#{item.id}
</foreach>
</update>
```
其中,动态 SQL 部分根据传入的参数来决定是否更新对应的字段。
2. 编写代码
```csharp
List<User> userList = new List<User>();
// 假设有三条数据需要更新
userList.Add(new User { id = 1, name = "John", age = 25, address = "New York" });
userList.Add(new User { id = 2, name = "Lucy", age = 28, address = "London" });
userList.Add(new User { id = 3, name = "Tom", age = 30 });
// 获取 SqlMapper 实例
ISqlMapper sqlMapper = Mapper.Instance();
// 开始事务
sqlMapper.BeginTransaction();
try
{
// 调用 SQL 语句更新数据
sqlMapper.Update("updateBatch", userList);
// 提交事务
sqlMapper.CommitTransaction();
}
catch(Exception ex)
{
// 回滚事务
sqlMapper.RollBackTransaction();
throw ex;
}
```
其中,User 类为要更新的数据类型,Mapper.Instance() 获取 SqlMapper 实例,updateBatch 为定义的 SQL 语句 ID。在 try-catch 块中执行 SQL 语句,如果出现异常则回滚事务,否则提交事务。
相关推荐
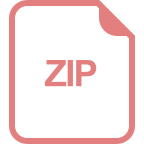














