void bubbleSort(ElemType list[], int len) { int temp; int flag=1; for (int index = 0; index < len && flag==1 ; ++index) { flag=0; for (int idx = 1; (1) ; idx++) { if (list[idx] < list[idx - 1]) { temp = list[idx]; list[idx] = list[idx - 1]; (2) ; } } } }补全代码
时间: 2024-02-15 07:12:27 浏览: 58
这段代码中缺少循环条件,应该是:
```
void bubbleSort(ElemType list[], int len) {
int temp;
int flag=1;
for (int index = 0; index < len && flag==1 ; ++index) {
flag=0;
for (int idx = 1; idx < len - index; idx++) {
if (list[idx] < list[idx - 1]) {
temp = list[idx];
list[idx] = list[idx - 1];
list[idx - 1] = temp;
flag=1;
}
}
}
}
```
这是一个冒泡排序的实现,用于对数组 `list` 进行排序,排序的长度为 `len`。
相关问题
补全下列冒泡排序代码 void bubbleSort(ElemType list[], int len) { int temp; int flag=1; for (int index = 0; index < len && flag==1 ; ++index) { flag=0; for (int idx = 1; (1) ; idx++) { if (list[idx] < list[idx - 1]) { temp = list[idx]; list[idx] = list[idx - 1]; (2) ; } } } }
void bubbleSort(ElemType list[], int len) {
int temp;
int flag=1;
for (int index = 0; index < len && flag==1 ; ++index) {
flag=0;
for (int idx = 1; idx < len - index; idx++) { //(1)补充代码
if (list[idx] < list[idx - 1]) {
temp = list[idx];
list[idx] = list[idx - 1];
list[idx - 1] = temp; //(2)补充代码
flag = 1;
}
}
}
}
void bubbleSort(int arr[], int n)
这是一个使用冒泡排序算法对整型数组 arr 进行升序排序的函数,其中 n 表示数组的长度。
函数实现如下:
```c++
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
```
冒泡排序的基本思路是:从数组的第一个元素开始,依次比较相邻的两个元素,如果前面的元素比后面的元素大,则交换它们的位置。经过一轮比较后,最大的元素会被移到末尾。然后再从第一个元素开始,重复上述操作,直到所有元素都被排好序为止。
阅读全文
相关推荐
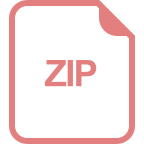
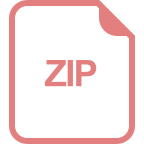
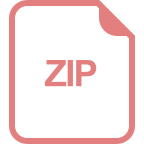














